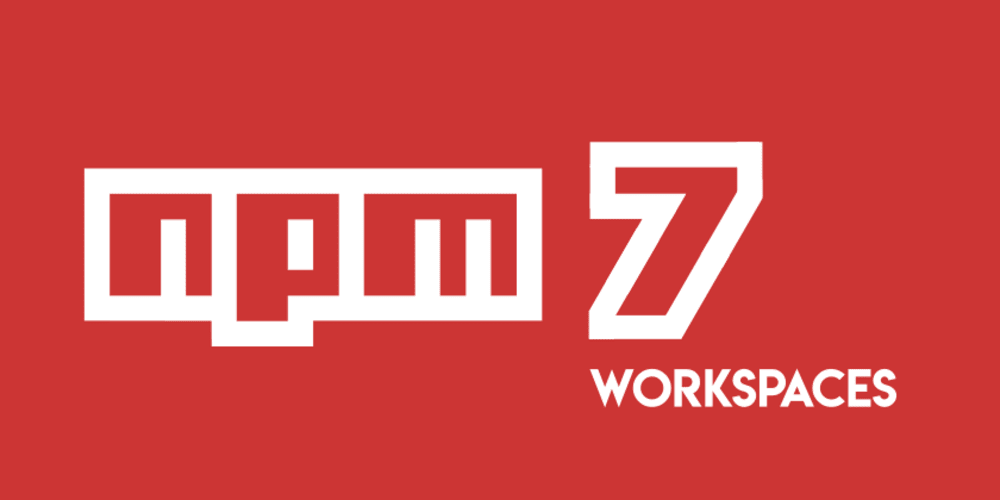
Unlock Shared Dependencies & More: npm Workspaces Explained
Introduction
npm workspaces are a powerful feature introduced in npm v7 that allows developers to manage multiple packages within a single repository. This feature is especially useful for monorepo setups where you might want to have multiple related packages managed in a cohesive way.
What Are npm Workspaces?
npm workspaces enable developers to manage multiple npm packages in a single repository. This setup is advantageous for projects where different packages are closely related and need to be developed and maintained together. Each package within the workspace can have its dependencies and be published separately, but they share a common node_modules folder, which helps in deduplication and consistency.
Setting Up npm Workspaces
To set up npm workspaces, you need to define them in your root package.json
file. Here is an example structure:
my-monorepo/
├── package.json
├── packages/
│ ├── package-a/
│ │ ├── package.json
│ ├── package-b/
│ │ ├── package.json
Root package.json
:
{
"name": "my-monorepo",
"version": "1.0.0",
"private": true,
"workspaces": [
"packages/*"
]
}
Each sub-package also has its own package.json
:
packages/package-a/package.json
:
{
"name": "package-a",
"version": "1.0.0",
"main": "index.js",
"dependencies": {
"lodash": "^4.17.21"
}
}
packages/package-b/package.json
:
{
"name": "package-b",
"version": "1.0.0",
"main": "index.js",
"dependencies": {
"react": "^17.0.2"
}
}
Pros of npm Workspaces
- Centralized Dependency Management:
Workspaces allow for a centralized management of dependencies, which can be shared across multiple packages. This ensures that all packages are using the same version of a dependency, reducing the risk of version conflicts.- Example:
Suppose bothpackage-a
andpackage-b
depend onlodash
. With workspaces, a single version oflodash
will be installed in the rootnode_modules
directory, avoiding duplication.
- Example:
{
"name": "my-monorepo",
"version": "1.0.0",
"private": true,
"workspaces": [
"packages/*"
],
"dependencies": {
"lodash": "^4.17.21"
}
}
- Simplified Development Workflow:
Workspaces streamline the development workflow by enabling you to run commands across multiple packages from the root level. For instance, you can runnpm install
to install dependencies for all packages ornpm run build
to build all packages.- Example:
{
"scripts": {
"build": "lerna run build"
}
}
- Efficient CI/CD Pipelines:
With workspaces, CI/CD pipelines can be configured more efficiently. Since dependencies are shared and deduplicated, builds are faster and require less storage space.- Example:
A CI configuration that installs all dependencies at once and runs tests for each package.
- Example:
jobs:
install:
steps:
- run: npm install
- run: npm run test --workspaces
- Modularization:
Workspaces encourage a modular architecture, making it easier to maintain and test individual components of a larger application. Each package can be developed, tested, and deployed independently.- Example:
Ifpackage-a
is a utility library andpackage-b
is a web application, they can be developed and tested independently, but still be part of the same repository.
- Example:
- Inter-package Dependency Management:
npm workspaces facilitate managing inter-package dependencies. Ifpackage-b
depends onpackage-a
, this relationship can be easily managed and updated within the workspace.- Example:
{
"dependencies": {
"package-a": "workspace:*"
}
}
Cons of npm Workspaces
- Complexity in Large Projects:
While workspaces simplify dependency management, they can add complexity to large projects with numerous packages. The more packages and interdependencies you have, the more challenging it can be to manage them effectively.- Example:
A monorepo with dozens of packages may require additional tools like Lerna or NX to manage the complexity.
- Example:
- Tooling Compatibility:
Not all tools in the JavaScript ecosystem fully support npm workspaces yet. Some tools and plugins may need additional configuration to work properly in a workspace environment.- Example:
Certain webpack configurations or plugins might need adjustment to resolve modules correctly in a workspace setup.
- Example:
- Dependency Hoisting Issues:
Hoisting can sometimes cause issues where dependencies are not placed where you expect them. This can lead to runtime errors if a package expects a dependency to be in its ownnode_modules
folder.- Example:
A package may not find its dependency if it assumes a localnode_modules
structure that has been hoisted to the root.
- Example:
- Versioning and Publishing Complexity:
Managing versions and publishing multiple packages can become complex, especially if you need to synchronize versions across multiple packages. Tools like Lerna can help, but they add another layer of complexity.- Example:
lerna publish
- Initial Learning Curve:
There is an initial learning curve associated with setting up and using npm workspaces, especially for teams that are not familiar with monorepo architectures.
Real-World Examples
Example 1: Building a Design System
A design system might consist of multiple packages for different components, utilities, and styles. Using npm workspaces, each component can be a separate package within the same repository.
Structure:
design-system/
├── package.json
├── packages/
│ ├── button/
│ │ ├── package.json
│ │ ├── Button.js
│ ├── input/
│ │ ├── package.json
│ │ ├── Input.js
│ ├── utils/
│ │ ├── package.json
│ │ ├── utils.js
Root package.json
:
{
"name": "design-system",
"version": "1.0.0",
"private": true,
"workspaces": [
"packages/*"
],
"scripts": {
"build": "lerna run build",
"test": "lerna run test"
},
"devDependencies": {
"lerna": "^4.0.0"
}
}
Button Package package.json
:
{
"name": "button",
"version": "1.0.0",
"main": "Button.js",
"dependencies": {
"react": "^17.0.2"
}
}
Example 2: Managing Microservices
A project with multiple microservices can also benefit from npm workspaces. Each microservice can be a separate package within the same repository.
Structure:
microservices/
├── package.json
├── services/
│ ├── auth-service/
│ │ ├── package.json
│ │ ├── index.js
│ ├── user-service/
│ │ ├── package.json
│ │ ├── index.js
Root package.json
:
{
"name": "microservices",
"version": "1.0.0",
"private": true,
"workspaces": [
"services/*"
],
"scripts": {
"start": "lerna run start",
"test": "lerna run test"
},
"devDependencies": {
"lerna": "^4.0.0"
}
}
Auth Service package.json
:
{
"name": "auth-service",
"version": "1.0.0",
"main": "index.js",
"dependencies": {
"express": "^4.17.1"
}
}
Conclusion
npm workspaces provide a robust and efficient way to manage multiple related packages within a single repository. They offer numerous benefits such as centralized dependency management, streamlined development workflows, and modularization. However, they also come with challenges like increased complexity in large projects, tooling compatibility issues, and an initial learning curve.
For projects that require a high degree of modularity and have multiple interrelated packages, npm workspaces can be an excellent choice. By leveraging workspaces, developers can maintain a clean and efficient monorepo setup, enabling faster development cycles and more maintainable codebases.
References
These resources provide additional insights and detailed information on setting up and managing workspaces effectively