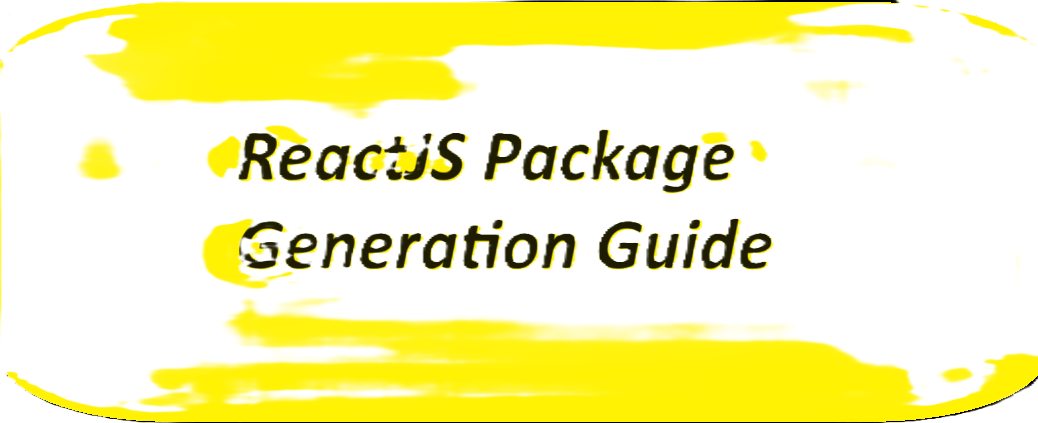
Building Your First ReactJS Package: A Complete Guide
Creating a ReactJS Package involves several steps, from setting up your development environment to publishing your package to a repository like npm. This guide will take you through the entire process, ensuring you have a clear understanding of each step. We’ll cover:
- Setting Up Your Development Environment
- Creating Your React Component
- Configuring Your Package
- Building Your Package
- Testing Your Package Locally
- Publishing Your Package to npm
- Using Your Package in a React Application
- Maintaining and Updating Your Package
1. Setting Up Your Development Environment
Before you begin, make sure you have Node.js and npm (Node Package Manager) installed on your system. You can download and install them from Node.js official website.
Create a New Directory
Start by creating a new directory for your package:
mkdir my-react-package
cd my-react-package
Initialize a New npm Package
Initialize a new npm package by running:
npm init -y
This command will create a package.json
file with default settings.
Install Required Dependencies
You’ll need several dependencies to create a React package. Install React, ReactDOM, and Babel:
npm install react react-dom
npm install --save-dev @babel/core @babel/cli @babel/preset-env @babel/preset-react
Set Up Babel Configuration
Create a .babelrc
file in the root directory of your project with the following content:
{
"presets": ["@babel/preset-env", "@babel/preset-react"]
}
2. Creating Your React Component
Next, create the React component that you want to package.
Create a Source Directory
Create a src
directory and inside it, create a file called index.js
:
mkdir src
touch src/index.js
Write Your React Component
Open src/index.js
and add the following code to create a simple React component:
import React from 'react';
const MyComponent = () => {
return (
<div>
<h1>Hello from MyComponent!</h1>
</div>
);
};
export default MyComponent;
3. Configuring Your Package
You need to update the package.json
file to configure your package correctly.
Update package.json
Open package.json
and update it to include the following properties:
{
"name": "my-react-package",
"version": "1.0.0",
"description": "A sample React component package",
"main": "dist/index.js",
"scripts": {
"build": "babel src -d dist"
},
"keywords": [
"react",
"component",
"package"
],
"author": "Your Name",
"license": "MIT",
"peerDependencies": {
"react": "^16.8.0 || ^17.0.0 || ^18.0.0",
"react-dom": "^16.8.0 || ^17.0.0 || ^18.0.0"
},
"devDependencies": {
"@babel/cli": "^7.14.5",
"@babel/core": "^7.14.6",
"@babel/preset-env": "^7.14.5",
"@babel/preset-react": "^7.14.5",
"react": "^17.0.2",
"react-dom": "^17.0.2"
}
}
4. Building Your Package
To build your package, you need to transpile your ES6+ React code into a format that can be consumed by other projects.
Create the Build Script
The build script defined in the package.json
uses Babel to transpile the code in the src
directory and output it to the dist
directory.
Run the build script:
npm run build
This command will generate the dist
directory containing the transpiled JavaScript file.
5. Testing Your Package Locally
Before publishing your package, it’s a good idea to test it locally to ensure everything works correctly.
Link Your Package
You can use npm link to link your package locally:
cd my-react-package
npm link
In another project where you want to test the package, run:
npm link my-react-package
Use Your Component in a React App
Create a new React app using create-react-app
(if you don’t already have one):
npx create-react-app test-app
cd test-app
npm link my-react-package
In the test-app/src/App.js
, import and use your component:
import React from 'react';
import MyComponent from 'my-react-package';
function App() {
return (
<div className="App">
<MyComponent />
</div>
);
}
export default App;
Run your test app to see your component in action:
npm start
6. Publishing Your Package to npm
Once you’re satisfied that your package works correctly, you can publish it to npm.
Log In to npm
If you haven’t logged in to npm before, run:
npm login
Publish Your Package
Publish your package to npm:
npm publish
7. Using Your Package in a React Application
Now that your package is published, you can use it in any React application by installing it from npm.
Install the Package
In any React project, install your package:
npm install my-react-package
Use Your Component
Import and use your component as you did in the test app:
import React from 'react';
import MyComponent from 'my-react-package';
function App() {
return (
<div className="App">
<MyComponent />
</div>
);
}
export default App;
8. Maintaining and Updating Your Package
After publishing your package, you might need to make updates or fix bugs. The process is straightforward:
Make Changes to Your Code
Edit the files in the src
directory as needed. After making changes, rebuild the package:
npm run build
Update the Version
Update the version number in package.json
according to semantic versioning rules (major.minor.patch):
"version": "1.0.1"
Publish the Updated Package
Publish the updated package:
npm publish
Conclusion
Creating a React package and publishing it to npm involves several steps, but by following this guide, you should be able to create, configure, build, test, and publish your package successfully. Here’s a quick recap of the steps:
- Set Up Your Development Environment: Install Node.js, npm, and necessary dependencies.
- Create Your React Component: Write your component code.
- Configure Your Package: Update
package.json
with the correct metadata and dependencies. - Build Your Package: Use Babel to transpile your code.
- Test Your Package Locally: Use
npm link
to test your package in a local project. - Publish Your Package to npm: Log in to npm and publish your package.
- Use Your Package: Install and use your package in any React application.
- Maintain and Update Your Package: Make changes, update the version, and republish as needed.
By understanding and following these steps, you’ll be able to create robust and reusable React components, making it easier to share your work with the developer community.