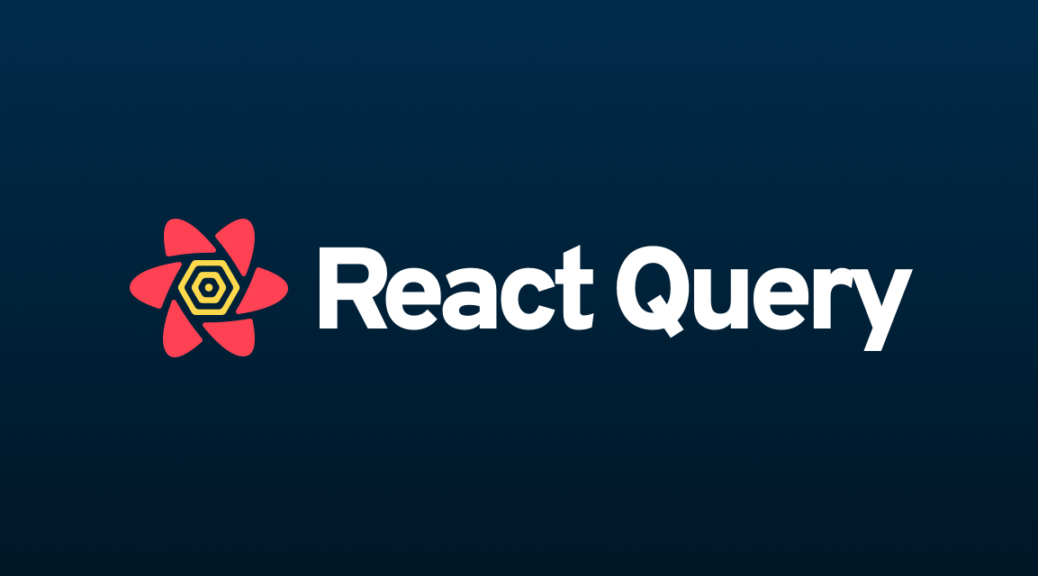
TanStack Query With Its Key Hooks and Examples
TanStack Query in a Nutshell:
- A powerful data fetching and caching library for React, Vue, Solid, Svelte, and vanilla JS.
- Simplifies asynchronous data management and reduces boilerplate code.
- Offers optimistic updates, automatic refetching, and offline support.
- Integrates seamlessly with React Query Devtools for debugging and inspection.
Key Hooks:
1. useQuery:
- Fetches data from an asynchronous source (API, database, etc.).
- Automatically caches and refetches data based on configuration.
- Manages loading, error, and success states.
Example:
JavaScript
import { useQuery } from '@tanstack/react-query';
function Posts() {
const { isLoading, error, data } = useQuery('posts', fetchPosts);
if (isLoading) return <div>Loading posts...</div>;
if (error) return <div>Error fetching posts: {error.message}</div>;
return (
<ul>
{data.map((post) => (
<li key={post.id}>{post.title}</li>
))}
</ul>
);
}
2. useInfiniteQuery:
- Fetches data in infinite scrolling or pagination scenarios.
- Fetches additional data as the user scrolls or navigates.
Example:
JavaScript
import { useInfiniteQuery } from '@tanstack/react-query';
function InfinitePosts() {
const { fetchNextPage, hasNextPage, isLoading, error, data } = useInfiniteQuery(
'posts',
fetchPosts,
{ getNextPageParam: (lastPage) => lastPage.nextPage }
);
// ... handle loading, error, and render posts
}
3. useMutation:
- Executes mutations (create, update, delete) on remote data.
- Manages optimistic updates and potentially refetches related queries.
Example:
JavaScript
import { useMutation } from '@tanstack/react-query';
function CreatePost() {
const createPostMutation = useMutation(createPost);
// ... handle form submission and mutation execution
}
Additional Hooks:
- useQueryClient: Access the query client for advanced control and custom hooks.
- useIsFetching: Check if a query is currently fetching data.
- useQueries: Fetch multiple queries in parallel.
Key Features:
- Optimistic Updates: Updates the UI immediately, even before server responses.
- Automatic Refetching: Refetches queries based on configuration (e.g., on focus, window focus, interval).
- Offline Support: Caches data for offline availability.
- React Query Devtools: Powerful debugging and inspection tool.
Official Resources:
- TanStack Query Documentation: https://tanstack.com/query (comprehensive overview, hooks, features, and examples)
- TanStack Query GitHub Repository: https://github.com/tanstack/query (source code, issues, and discussions)
- TanStack Query Devtools: https://chrome.google.com/webstore/