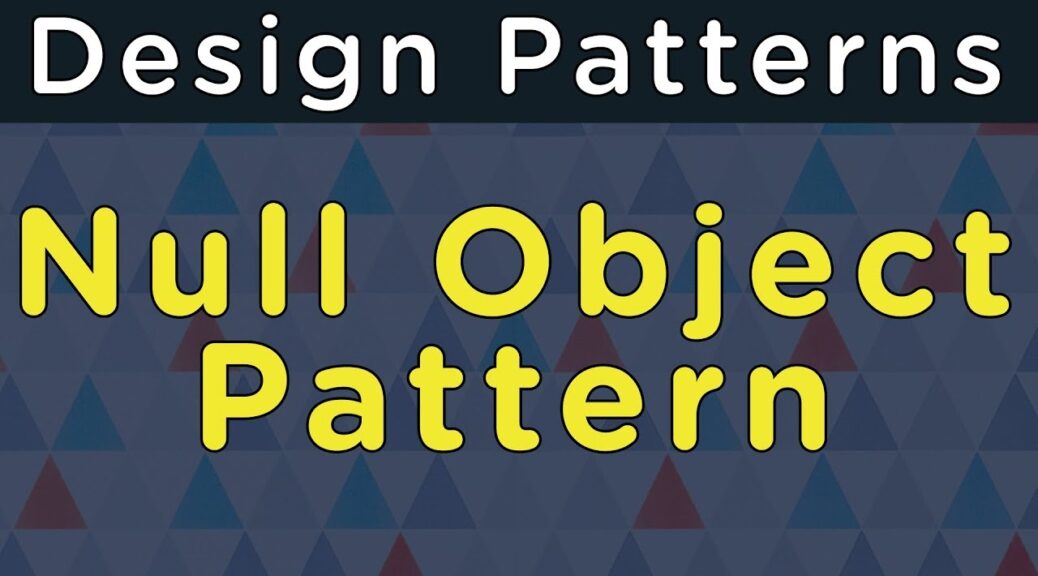
How to Implement the Null Object Design Pattern in .NET Core
The Null Object Design Pattern is a behavioral design pattern that provides a default object as a surrogate for the absence of a value or object, avoiding the need to check for null values. In the context of .NET Core, this pattern can be especially useful for avoiding null reference exceptions and simplifying code.
Implementation Steps
1. Define an Interface or Abstract Class
Start by defining an interface or abstract class that the real object and the null object will implement.
public interface ICustomer
{
void Purchase();
}
2. Implement the Real Object
Create a concrete implementation of the interface or abstract class for the actual object.
public class RealCustomer : ICustomer
{
public void Purchase()
{
Console.WriteLine("Customer made a purchase.");
}
}
3. Implement the Null Object
Create a concrete implementation of the interface or abstract class for the null object, which will have no meaningful behavior.
public class NullCustomer : ICustomer
{
public void Purchase()
{
// Do nothing
}
}
4. Modify the Factory or Method to Return Null Object
Ensure that the code responsible for returning instances of the object will return a Null Object instead of null.
public class CustomerFactory
{
public static ICustomer GetCustomer(string customerId)
{
if (string.IsNullOrEmpty(customerId))
{
return new NullCustomer();
}
// Logic to retrieve a real customer based on customerId
return new RealCustomer();
}
}
5. Use the Objects Without Null Checks
Utilize the objects without checking for null, as the Null Object will handle the absence case.
public class ShoppingCart
{
private readonly ICustomer _customer;
public ShoppingCart(ICustomer customer)
{
_customer = customer;
}
public void CompletePurchase()
{
_customer.Purchase();
}
}
// Usage
var customer = CustomerFactory.GetCustomer(null); // Returns NullCustomer
var cart = new ShoppingCart(customer);
cart.CompletePurchase(); // Safe, no need for null checks
Pros and Cons
Pros
- Eliminates Null Checks: Simplifies code by removing the need to check for null values.
- Reduces Null Reference Exceptions: Minimizes the risk of encountering null reference exceptions.
- Adheres to Open/Closed Principle: Allows the system to be extended with new behavior without modifying existing code.
- Improves Readability: Makes the code more readable and maintainable by clearly defining the default behavior.
Cons
- Potential for Misuse: Overuse of the Null Object Pattern can lead to hidden errors where the absence of action is significant.
- Overhead: May introduce unnecessary objects, potentially impacting performance if overused in performance-critical sections.
- Complexity: Adds an additional layer of abstraction, which may complicate understanding for developers not familiar with the pattern.
- Semantics: The Null Object might hide the fact that something important is missing, leading to bugs that are harder to trace.
Conclusion
The Null Object Design Pattern is a powerful tool in .NET Core for managing the absence of objects in a clean and maintainable way. By returning a Null Object instead of null, it avoids null reference exceptions and simplifies client code. However, it should be used judiciously to avoid potential pitfalls such as hidden errors and unnecessary complexity.