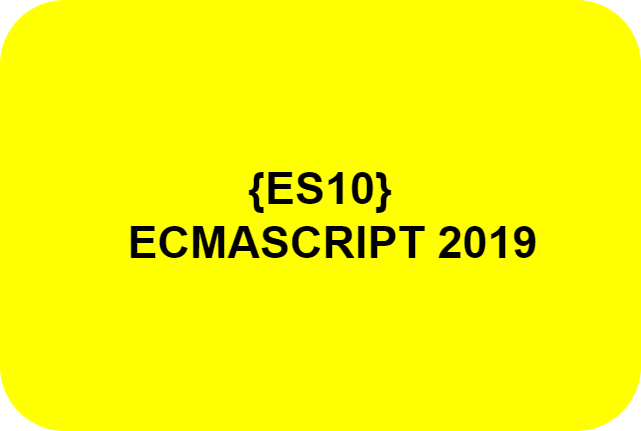
Master JavaScript’s Latest Updates: A Guide to ES10 (ECMAScript 2019):
Here are key features introduced in ES10 (ECMAScript 2019) with detailed descriptions and examples:
1. Array.flat() and Array.flatMap():
- Array.flat(): Flattens nested arrays into a single, shallower array.
JavaScript
const nestedArray = [[1, 2], [3, 4]];
const flattened = nestedArray.flat(); // [1, 2, 3, 4]
- Array.flatMap(): Combines mapping and flattening in one operation.
JavaScript
const numbers = [1, 2, 3];
const doubled = numbers.flatMap(x => [x, x * 2]); // [1, 2, 2, 4, 3, 6]
2. Object.fromEntries():
- Creates an object from a list of key-value pairs (arrays).
JavaScript
const entries = [["name", "John"], ["age", 30]];
const person = Object.fromEntries(entries); // { name: "John", age: 30 }
3. String.trimStart() and String.trimEnd():
- Remove whitespace from the beginning or end of strings.
JavaScript
const str = " Hello World! ";
const trimmedStart = str.trimStart(); // "Hello World! "
const trimmedEnd = str.trimEnd(); // " Hello World!"
4. Symbol.description:
- Provides a human-readable description for Symbols, useful for debugging.
JavaScript
const mySymbol = Symbol("foo");
mySymbol.description = "My special symbol";
console.log(mySymbol.description); // "My special symbol"
5. Optional Catch Binding:
- Eliminates the need to define an unused error variable in
catch
blocks.
JavaScript
try {
// ...
} catch {
// Handle error without explicit error variable
}
6. JSON Superset:
- Supports parsing JSON with JavaScript-like syntax, including unquoted property names and trailing commas.
JavaScript
const parsed = JSON.parse('{ a: 1, b: 2, }'); // Valid JSON
7. BigInt Primitive:
- Represents arbitrarily large integers beyond the safe integer limit of JavaScript numbers.
JavaScript
const bigNumber = 9007199254740991n; // BigInt literal
8. Dynamic Import:
- Loads modules dynamically at runtime using
import()
.
JavaScript
const module = await import('./myModule.js');
Note: These features continue JavaScript’s evolution, offering more concise syntax, enhanced array and object manipulation, error handling, and data representation capabilities.