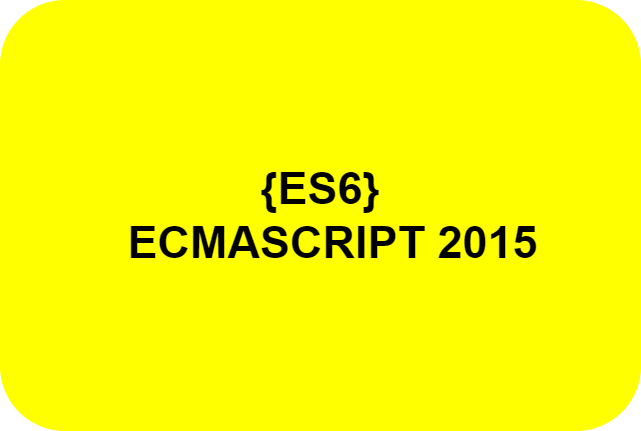
Master JavaScript’s Latest Updates: A Guide to ES6 (ECMASCRIPT 2015):
Here are the key features introduced in ES6 (ECMAScript 2015) with detailed descriptions and examples:
1. Block-Scoped Variables (let and const):
let: Declares variables with block scope, meaning they’re only accessible within the block where they’re declared (e.g., within an if
statement or a loop).
JavaScript
if (true) {
let x = 10;
}
console.log(x); // ReferenceError: x is not defined
const: Declares variables that cannot be reassigned after their initial assignment.
JavaScript
const PI = 3.14159;
PI = 2.5; // Error: Assignment to constant variable
2. Arrow Functions:
Concise way to write functions with implicit this
binding and optional return expressions.
JavaScript
const add = (a, b) => a + b;
const greet = (name) => console.log(`Hello, ${name}!`);
3. Template Literals:
Tagged templates for string interpolation and multi-line strings.
JavaScript
const name = "John";
const age = 30;
const message = `Hello, ${name}! You are ${age} years old.`;
4. Destructuring:
Extract values from arrays and objects into distinct variables.
JavaScript
const person = { name: "Alice", age: 30 };
const { name, age } = person;
5. Classes:
Blueprints for creating objects with a more structured approach.
JavaScript
class Person {
constructor(name, age) {
this.name = name;
this.age = age;
}
greet() {
console.log(`Hello, my name is ${this.name}!`);
}
}
6. Modules:
System for organizing code into reusable modules.
JavaScript
// math.js
export const PI = 3.14159;
export function square(x) {
return x * x;
}
7. Default Parameters:
Assign default values to function parameters.
JavaScript
function greet(name = "World") {
console.log(`Hello, ${name}!`);
}
8. Rest Parameter (…):
Collects remaining arguments into an array.
JavaScript
function sum(...numbers) {
return numbers.reduce((total, num) => total + num, 0);
}
9. Spread Operator (…):
Expands iterables into individual elements.
JavaScript
const numbers = [1, 2, 3];
const newNumbers = [...numbers, 4, 5];
10. Promises:
Handle asynchronous operations more elegantly.
JavaScript
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
Learning Resources:
If you’re interested in learning more about ES6, here are some helpful resources:
- Mozilla Developer Network: https://developer.mozilla.org/en-US/docs/Web/JavaScript
- JavaScript ES6 Tutorial: https://www.programiz.com/javascript/ES6
- Interactive ES6 Tutorial: https://support.khanacademy.org/hc/en-us/community/posts/4418180684045-JavaScript-ES6-support-MUST-be-implemented-