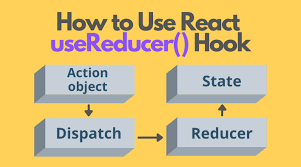
Reducer With Great Detail In ReactJS:
What is a Reducer?
- A reducer is a pure function that takes the current state of an application and an action object, and returns the new state.
- It’s responsible for handling state updates in a predictable and centralized way.
- It’s often used with the
useReducer
hook in React, or as a core concept in state management libraries like Redux.
How It Works:
- Action Dispatched: When an event occurs (e.g., button click, data fetch), an action object is dispatched, describing the intended state change.
- Reducer Called: The reducer receives the current state and the action object.
- New State Calculated: The reducer, based on the action type and state, computes the new state immutably.
- State Updated: The component’s state is updated with the new state returned by the reducer.
- Component Re-renders: React re-renders the component with the updated state.
Example:
JavaScript
import React, { useReducer } from 'react';
function todoReducer(state, action) {
switch (action.type) {
case 'ADD_TODO':
return [...state, action.payload];
case 'TOGGLE_TODO':
return state.map((todo) =>
todo.id === action.payload.id ? { ...todo, completed: !todo.completed } : todo
);
default:
return state;
}
}
function TodoList() {
const [todos, dispatch] = useReducer(todoReducer, []);
// ... rest of the component
}
Pros:
- Centralized State Management: Reducers consolidate state logic, making it easier to manage and understand.
- Predictable Updates: State updates are deterministic, making debugging and reasoning about changes simpler.
- Testability: Reducers, as pure functions, are straightforward to test in isolation.
- Complex State Handling: Reducers excel at managing intricate state relationships and dependencies.
- Scalability: Reducers can be combined and composed to manage large applications effectively.
Cons:
- Boilerplate: For simple states,
useReducer
might introduce more code thanuseState
. - Learning Curve: Understanding reducers and their patterns requires some initial effort.
- Overkill for Simple Cases: Not always necessary for straightforward state management.
Best Practices:
- Keep reducers pure and avoid side effects.
- Use clear and concise action types.
- Structure reducers for readability and maintainability.
- Combine reducers for complex state using techniques like
combineReducers
. - Consider using a state management library like Redux for large-scale applications.