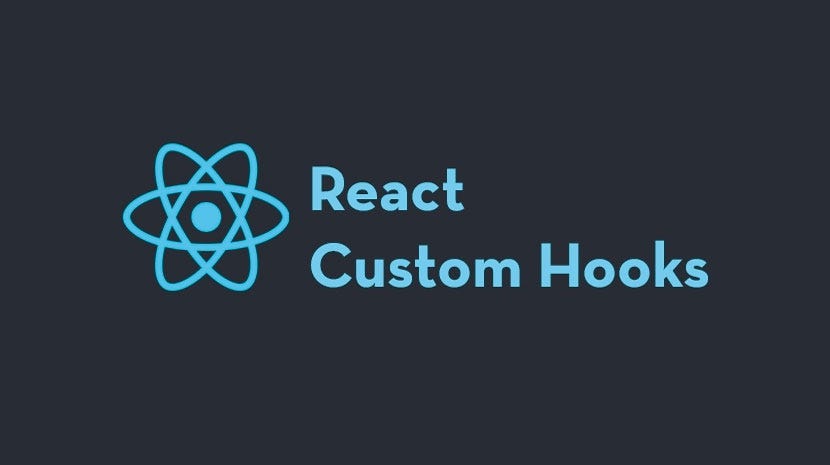
How to create custom hook in React JS:
1. Create a New File:
- Start by creating a new JavaScript file to house your custom hook. Naming conventions typically use the prefix
use
, likeuseCountdown.js
oruseWindowSize.js
.
2. Define the Hook Function:
- Within the file, define a function that represents your custom hook. Adhere to the naming convention by starting the function name with
use
. - Inside the function, you can:
- Utilize built-in React hooks like
useState
,useEffect
,useContext
, etc. - Perform any necessary logic or computations.
- Return the values or functions you want to expose to components.
- Utilize built-in React hooks like
3. Set Up Inputs and Outputs:
- Decide what inputs your hook requires and what outputs it should provide.
- Declare input parameters for the function.
- Return the necessary values or functions from the hook.
4. Use Built-in Hooks:
- Compose your custom hook using built-in React hooks.
- For example, you might use
useState
to manage state within the hook oruseEffect
to perform side effects.
5. Export the Hook:
- Export the hook function from the file so it can be imported and used in other components.
Example: Creating a useCountdown
Hook:
JavaScript
import React, { useState, useEffect } from 'react';
function useCountdown(duration) {
const [secondsRemaining, setSecondsRemaining] = useState(duration);
useEffect(() => {
const intervalId = setInterval(() => {
setSecondsRemaining((prevSeconds) => prevSeconds - 1);
}, 1000);
return () => clearInterval(intervalId); // Cleanup function
}, [duration]);
return secondsRemaining;
}
export default useCountdown;
Using the Custom Hook in a Component:
JavaScript
import React from 'react';
import useCountdown from './useCountdown';
function MyComponent() {
const seconds = useCountdown(10);
return (
<div>
Time remaining: {seconds} seconds
</div>
);
}
Key Points:
- Custom hooks enhance code reusability, organization, and testability.
- They encapsulate stateful logic and side effects, making components cleaner.
- Follow the naming convention (
use
) to distinguish them from regular functions. - Only call hooks at the top level of React components, not inside loops or conditionals.