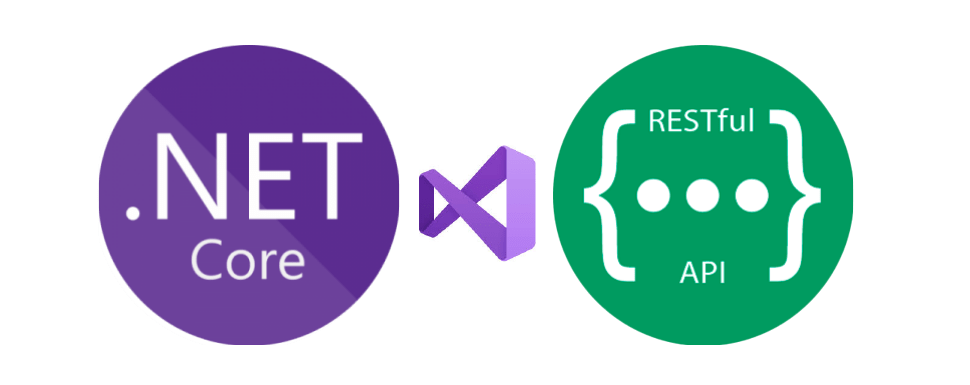
ASP.NET Core Web API Components with Examples: A Quick Guide
ASP.NET Core Web API is a framework provided by Microsoft for building scalable, high-performance web APIs on the .NET platform. It is a part of the ASP.NET Core suite, which is an open-source, cross-platform framework for building modern, cloud-based, and internet-connected applications. ASP.NET Core Web API enables developers to create RESTful services that can be consumed by a variety of clients, including web browsers, mobile devices, and other servers.
Here is a detailed explanation key components in an ASP.NET Core Web API, with examples:
1. Controller
Controller in ASP.NET Core Web API is a class that handles HTTP requests and generates HTTP responses. It serves as the entry point for handling client requests.
Example:
using Microsoft.AspNetCore.Mvc;
[ApiController]
[Route("api/[controller]")]
public class WeatherController : ControllerBase
{
[HttpGet]
public IActionResult Get()
{
return Ok(new { Temperature = 25, Condition = "Sunny" });
}
}
In this example, WeatherController
responds to GET requests to api/weather
and returns a JSON response with the weather information.
2. Routing
Routing defines how URL paths are mapped to controller actions. It is configured in the Startup.cs
file or via attributes on controllers and actions.
Example:
app.UseEndpoints(endpoints =>
{
endpoints.MapControllers();
});
In the controller:
[Route("api/weather")]
public class WeatherController : ControllerBase
{
[HttpGet("{city}")]
public IActionResult Get(string city)
{
// Implementation here
}
}
This setup maps GET requests to api/weather/{city}
to the Get
method in WeatherController
.
3. Versioning
Versioning is used to manage different versions of an API. This allows for backward compatibility and gradual upgrades.
Example:
First, install the versioning package:
dotnet add package Microsoft.AspNetCore.Mvc.Versioning
In Startup.cs
:
services.AddApiVersioning(config =>
{
config.DefaultApiVersion = new ApiVersion(1, 0);
config.AssumeDefaultVersionWhenUnspecified = true;
config.ReportApiVersions = true;
});
In the controller:
[ApiVersion("1.0")]
[Route("api/v{version:apiVersion}/weather")]
public class WeatherV1Controller : ControllerBase
{
[HttpGet]
public IActionResult Get()
{
return Ok(new { Temperature = 25, Condition = "Sunny" });
}
}
[ApiVersion("2.0")]
[Route("api/v{version:apiVersion}/weather")]
public class WeatherV2Controller : ControllerBase
{
[HttpGet]
public IActionResult Get()
{
return Ok(new { Temperature = 22, Condition = "Cloudy", Humidity = 60 });
}
}
4. Middleware
Middleware components are used to handle requests and responses in the pipeline. They can process requests before they reach the controller or handle responses before they are sent to the client.
Example:
public class CustomMiddleware
{
private readonly RequestDelegate _next;
public CustomMiddleware(RequestDelegate next)
{
_next = next;
}
public async Task InvokeAsync(HttpContext context)
{
// Custom logic here
await _next(context);
// Custom logic here
}
}
In Startup.cs
:
app.UseMiddleware<CustomMiddleware>();
5. Attributes
Attributes provide a way to add metadata to code elements (like classes, methods) and control behavior like routing, validation, and authorization.
Example:
[ApiController]
[Route("api/[controller]")]
public class WeatherController : ControllerBase
{
[HttpGet]
[Authorize]
public IActionResult Get()
{
return Ok(new { Temperature = 25, Condition = "Sunny" });
}
}
Here, [Authorize]
is an attribute that restricts access to authenticated users only.
6. Filters
Filters are used to run code before or after certain stages in the request processing pipeline, such as authorization, action execution, or result execution.
Example:
public class CustomActionFilter : IActionFilter
{
public void OnActionExecuting(ActionExecutingContext context)
{
// Code before action execution
}
public void OnActionExecuted(ActionExecutedContext context)
{
// Code after action execution
}
}
In Startup.cs
:
services.AddControllers(config =>
{
config.Filters.Add<CustomActionFilter>();
});
7. Program.cs
Program.cs is the entry point for the application. It configures and starts the web host.
Example:
public class Program
{
public static void Main(string[] args)
{
CreateHostBuilder(args).Build().Run();
}
public static IHostBuilder CreateHostBuilder(string[] args) =>
Host.CreateDefaultBuilder(args)
.ConfigureWebHostDefaults(webBuilder =>
{
webBuilder.UseStartup<Startup>();
});
}
8. appsettings.json
appsettings.json is used to store configuration settings, such as connection strings, app settings, and environment-specific settings.
Example:
{
"Logging": {
"LogLevel": {
"Default": "Information",
"Microsoft": "Warning"
}
},
"AllowedHosts": "*",
"ConnectionStrings": {
"DefaultConnection": "Server=(localdb)\\mssqllocaldb;Database=aspnet-CoreApp;Trusted_Connection=True;MultipleActiveResultSets=true"
}
}
9. Dependency Injection
Dependency Injection (DI) is a technique to achieve Inversion of Control (IoC) between classes and their dependencies.
Example:
Define a service interface and implementation:
public interface IWeatherService
{
string GetWeather();
}
public class WeatherService : IWeatherService
{
public string GetWeather()
{
return "Sunny";
}
}
Register the service in Startup.cs
:
public void ConfigureServices(IServiceCollection services)
{
services.AddTransient<IWeatherService, WeatherService>();
services.AddControllers();
}
Use the service in a controller:
[ApiController]
[Route("api/[controller]")]
public class WeatherController : ControllerBase
{
private readonly IWeatherService _weatherService;
public WeatherController(IWeatherService weatherService)
{
_weatherService = weatherService;
}
[HttpGet]
public IActionResult Get()
{
var weather = _weatherService.GetWeather();
return Ok(new { Weather = weather });
}
}
10. Kestrel
Kestrel is a cross-platform web server for ASP.NET Core. It is the default web server and provides fast and efficient handling of HTTP requests.
Example:
Kestrel is typically configured in Program.cs
:
public static IHostBuilder CreateHostBuilder(string[] args) =>
Host.CreateDefaultBuilder(args)
.ConfigureWebHostDefaults(webBuilder =>
{
webBuilder.UseKestrel();
webBuilder.UseStartup<Startup>();
});
You can configure Kestrel in appsettings.json
:
"Kestrel": {
"Endpoints": {
"Http": {
"Url": "http://localhost:5000"
},
"Https": {
"Url": "https://localhost:5001",
"Certificate": {
"Path": "path/to/certificate.pfx",
"Password": "password"
}
}
}
}
These components form the core of an ASP.NET Core Web API, providing a robust framework for building web applications and services. Refer below links to explore more about ASP.NET Core Web API.
- ASP.NET Core Web API documentation: Comprehensive guide and tutorials on building Web APIs with ASP.NET Core.
- Create a web API with ASP.NET Core: Step-by-step learning module with exercises to build a Web API using ASP.NET Core.
- ASP.NET Core Samples: Official GitHub repository with samples for various ASP.NET Core scenarios.