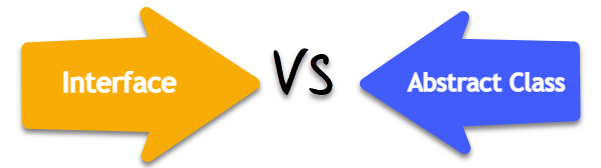
Abstract vs. Interface? Know the When & Why
Abstract classes and interfaces are both fundamental concepts in object-oriented programming that promote code reusability and flexibility. However, they serve distinct purposes:
Abstract Classes:
Purpose:
- Serve as blueprints for derived classes, defining a common foundation.
- Enforce implementation of specific behaviors through abstract methods.
- Offer shared functionality through concrete methods (optional).
Key Features:
- Can contain both abstract and concrete methods.
- Can have constructors and access modifiers (public, private, protected).
- Can hold fields and properties.expand_more
- Classes can only inherit from one abstract class directly.exclamation
Example:
C#:
public abstract class Animal
{
public abstract void MakeSound(); // Abstract method (must be implemented)
public virtual void Eat() // Concrete method (can be overridden)
{
Console.WriteLine("The animal is eating.");
}
}
Interfaces:
Purpose:
- Define contracts that classes must adhere to, ensuring consistency.
- Specify a set of functionalities that implementing classes must provide.
Key Features:
- Only contain abstract methods (no implementation).
- Cannot have constructors, fields, or properties.
- Classes can implement multiple interfaces.expand_more
Example:
C#:
public interface IShape
{
double CalculateArea(); // Abstract method
}
Key Differences Summary:
Feature | Abstract Class | Interface |
---|---|---|
Purpose | Blueprint for inheritance | Contract for behavior |
Methods | Abstract and concrete | Abstract only |
Inheritance | Single inheritance | Multiple inheritance |
Constructors | Can have constructors | No constructors |
Fields/Properties | Can have fields and properties | No fields or properties |
When to Use Which:
Abstract Classes:
- When you need to establish a common base for related classes with shared functionality.
- When you want to control implementation details for some methods while allowing flexibility for others.
Interfaces:
- When you want to define a contract across unrelated classes that share specific behaviors.
- When you need loose coupling and flexibility in class design.
- When classes need to adhere to multiple contracts (multiple interface implementation).
Choosing Between Abstract Class and Interface:
- Use an abstract class when you want to define a base class with shared functionalities (concrete methods) and force derived classes to implement specific behaviors (abstract methods).
- Use an interface when you want to define a set of functionalities that various unrelated classes can implement independently.
By understanding these distinctions, you can effectively leverage abstract classes and interfaces to create more robust, reusable, and maintainable object-oriented code in C#.
Here are some helpful resources related to the differences between abstract classes and interfaces in C#, including both explanations and examples:
Official Microsoft Documentation: