Introduction
OpenAI’s o1 sequence fashions signify a big leap in massive language mannequin (LLM) capabilities, notably for advanced reasoning duties. These fashions interact in deep inside thought processes earlier than responding, making them wonderful at fixing intricate issues in science, arithmetic, and programming. This text will information you thru the important thing options of the OpenAI o1 API, introduce the accessible fashions, and supply sensible examples of methods to use their superior reasoning and code era skills.
Key Options of the o1 Fashions
The o1 fashions excel at tackling issues requiring logical, scientific, and mathematical reasoning. They rank within the 89th percentile in aggressive programming (Codeforces) and surpass PhD-level accuracy in physics, biology, and chemistry benchmarks (GPQA). They’ve even positioned among the many high 500 college students within the US within the AIME qualifier for the USA Math Olympiad.
There are two fashions presently accessible:
- o1-preview: This mannequin supplies an early have a look at the total capabilities of o1, utilizing broad basic information to unravel advanced issues.
- o1-mini: A sooner, extra environment friendly model of the o1 mannequin, optimized for duties corresponding to coding, math, and scientific reasoning.
With restricted options, the o1 fashions at the moment are in beta testing. Solely builders in tier 5 are permitted entry, and there are low charge caps (20 RPM).
Additionally Learn: The right way to Entry OpenAI o1?
Pricing and Mannequin Specs for OpenAI o1-mini and o1-preview
OpenAI has launched two variants of the o1 mannequin sequence, every with totally different pricing and capabilities tailor-made to particular use circumstances:
OpenAI o1-mini
This mannequin is optimized for coding, math, and science duties, offering an economical answer for builders and researchers. It has a 128K context and makes use of the October 2023 information cutoff.
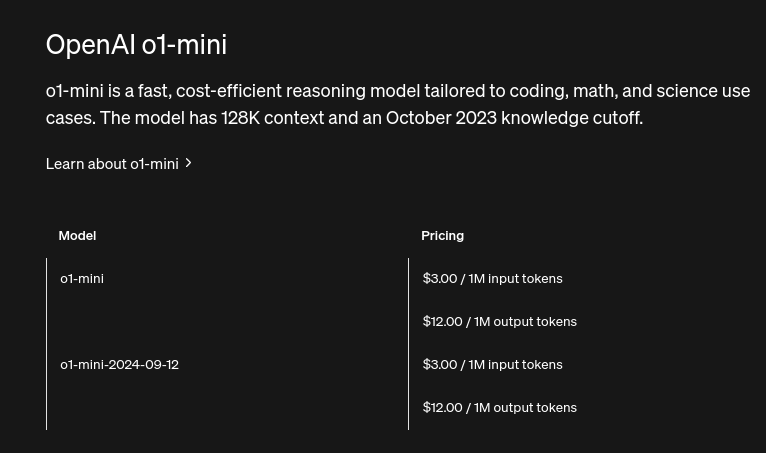
- Pricing: $3.00 per 1 million tokens.
- Output tokens: $12.00 per 1 million tokens.
OpenAI o1-preview
Designed for extra advanced duties that require broad basic information, the o1-preview mannequin is positioned for superior reasoning and problem-solving. It additionally has 128K context and attracts on the October 2023 information cutoff.
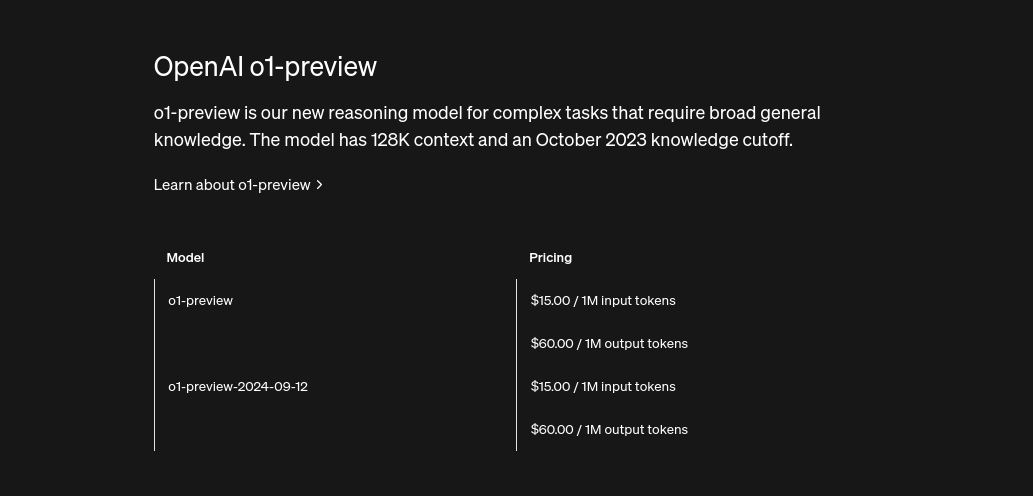
- Pricing: $15.00 per 1 million tokens.
- Output tokens: $60.00 per 1 million tokens.
o1-mini vs o1-preview
Function | o1-mini | o1-preview |
---|---|---|
Goal Viewers | Builders and researchers | Basic customers, professionals, and organizations |
Main Focus | Excessive reasoning energy in particular fields like coding and math | Basic information capabilities with deeper reasoning throughout a number of disciplines |
Price | Extra cost-efficient | Larger price |
Use Instances | Appropriate for duties requiring specialised reasoning, corresponding to coding or math | Ideally suited for dealing with advanced, multidisciplinary duties that require broad and deep information |
Efficiency Traits | Focuses on domain-specific experience to realize excessive accuracy and velocity | Emphasizes complete understanding and suppleness for varied advanced issues and inquiries |
Additionally Learn: GPT-4o vs OpenAI o1: Is the New OpenAI Mannequin Well worth the Hype?
The right way to Entry OpenAI o1 API?
Here’s a step-by-step information on methods to entry and use the OpenAI o1 API:
Step 1: Get API Entry
- Join API Entry: In case you are not already a part of the OpenAI beta program, you’ll have to request entry by visiting OpenAI’s API web page. When you join, you could want to attend for approval to entry the o1 fashions.
- Generate an API Key: Upon getting entry, log in to the OpenAI API platform and generate an API key. This key’s needed for making API requests.
- Go to API Keys and click on on “Create New Secret Key”.
- Copy the important thing and reserve it securely, as you’ll want it within the code examples.
Step 2: Set up the OpenAI Python SDK
To work together with the o1 API, you’ll need to put in the OpenAI Python SDK. You are able to do this utilizing the next command:
pip set up openai
This bundle permits you to make API requests to OpenAI out of your Python code.
Step 3: Initialize the OpenAI Consumer
When you’ve put in the SDK and obtained your API key, you possibly can initialize the shopper in Python as proven beneath:
from openai import OpenAI
# Initialize the OpenAI shopper together with your API key
shopper = OpenAI(api_key="your-api-key")
Exchange “your-api-key” with the precise API key you generated earlier.
Utilizing the o1 API for Code Era
Now that you simply’ve arrange your OpenAI shopper, let’s have a look at an instance the place we use the o1-preview mannequin to generate a Python perform that converts temperatures between Fahrenheit and Celsius.
Step 1: Craft the Immediate
On this instance, we are going to ask the mannequin to put in writing a Python perform that converts a temperature from Fahrenheit to Celsius and vice versa.
immediate = """
Write a Python perform that converts a temperature from Fahrenheit to Celsius and vice versa.
The perform ought to take an enter, decide the sort (Fahrenheit or Celsius), and return the transformed temperature.
"""
Step 2: Make the API Request
We’ll go this immediate to the o1 mannequin utilizing the chat.completions.create() technique, specifying the mannequin we need to use (o1-preview) and the person message.
response = shopper.chat.completions.create(
mannequin="o1-preview",
messages=[
{
"role": "user",
"content": prompt
}
]
)
# Output the generated Python code
print(response.selections[0].message.content material)
On this instance, the o1-preview mannequin intelligently handles the logic for temperature conversion, exhibiting its proficiency in fixing easy coding duties. Relying on the complexity, these requests could take just a few seconds or longer.
Output:
```python
def convert_temperature(temp_input):
"""
Converts a temperature from Fahrenheit to Celsius or vice versa.
Parameters:
temp_input (str): A temperature enter string, e.g., '100F' or '37C'.
Returns:
str: The transformed temperature with the unit.
"""
import re # Importing contained in the perform to maintain the scope native
# Take away main and trailing whitespaces
temp_input = temp_input.strip()
# Common expression to parse the enter string
match = re.match(r'^([+-]?[0-9]*.?[0-9]+)s*([cCfF])
Beta Limitations
Through the beta section, sure options of the o1 API will not be but totally supported. Key limitations embrace:
- Modalities: Textual content solely, no picture assist.
- Message Sorts: Solely person and assistant messages, no system messages.
- Streaming: Not supported.
- Instruments and Features: Not but accessible, together with response format parameters and performance calling.
- Temperature and Penalties: Mounted values for temperature, top_p, and penalties.
Coding and Reasoning with o1 Fashions
The o1 fashions excel at dealing with algorithmic duties and reasoning. Right here’s an up to date instance the place the o1-mini mannequin is tasked with discovering the sum of all prime numbers beneath 100:
Create the Immediate
Write a transparent immediate that describes the duty you need the mannequin to carry out. On this case, the duty is to put in writing a Python perform that calculates the sum of all prime numbers beneath 100:
immediate = """
Write a Python perform that calculates the sum of all prime numbers beneath 100.
The perform ought to first decide whether or not a quantity is prime, after which sum up
all of the prime numbers beneath 100.
"""
Make the API Name
Use the chat.completions.create technique to ship the immediate to the o1-mini mannequin. Right here’s the whole code:
response = shopper.chat.completions.create(
mannequin="o1-mini",
messages=[
{
"role": "user",
"content": prompt
}
]
)
print(response.selections[0].message.content material)
This instance reveals how the o1-mini mannequin is able to writing environment friendly code for duties like figuring out prime numbers and performing fundamental mathematical operations.
Output
Definitely! Beneath is a Python perform that calculates the sum of all prime numbers beneath 100. The perform features a helper perform `is_prime` to find out if a quantity is prime. After figuring out all prime numbers beneath 100, it sums them up and returns the whole.
```python
def is_prime(n):
"""
Examine if a quantity is a major quantity.
Parameters:
n (int): The quantity to examine for primality.
Returns:
bool: True if n is prime, False in any other case.
"""
if n < 2:
return False
# Solely have to examine as much as the sq. root of n
for i in vary(2, int(n**0.5) + 1):
if n % i == 0:
return False
return True
def sum_primes_below_100():
"""
Calculate the sum of all prime numbers beneath 100.
Returns:
int: The sum of prime numbers beneath 100.
"""
prime_sum = 0
for quantity in vary(2, 100):
if is_prime(quantity):
prime_sum += quantity
return prime_sum
# Name the perform and print the end result
whole = sum_primes_below_100()
print(f"The sum of all prime numbers beneath 100 is: {whole}")
```
### Rationalization:
1. **Helper Perform (`is_prime`)**:
- **Goal**: Determines whether or not a given quantity `n` is a major quantity.
- **Logic**:
- Numbers lower than 2 will not be prime.
- For numbers 2 and above, examine divisibility from 2 as much as the sq. root of `n`. If `n` is divisible by any of those numbers, it isn't prime.
- If no divisors are discovered, the quantity is prime.
2. **Essential Perform (`sum_primes_below_100`)**:
- **Goal**: Iterates by way of all numbers from 2 as much as (however not together with) 100.
- **Logic**:
- For every quantity within the vary, it makes use of the `is_prime` perform to examine if it is prime.
- If the quantity is prime, it is added to the cumulative `prime_sum`.
- **Return**: The entire sum of all prime numbers beneath 100.
3. **Execution**:
- The perform `sum_primes_below_100` known as, and the result's saved within the variable `whole`.
- The sum is then printed to the console.
### Output
If you run the above code, it's going to output:
```
The sum of all prime numbers beneath 100 is: 1060
```
Which means that the sum of all prime numbers beneath 100 is **1060**.
Extra Complicated Use Instances
The o1-preview mannequin can deal with reasoning-heavy duties, corresponding to outlining a plan for growing a list administration system. Beneath is an instance the place the mannequin supplies a high-level construction for the venture:
Create Immediate
immediate = """
I need to develop a list administration system that tracks merchandise, portions,
and places. It ought to notify the person when inventory is low. Create a plan for the
listing construction and supply code snippets for the important thing elements.
"""
Make API Name
response = shopper.chat.completions.create(
mannequin="o1-preview",
messages=[
{
"role": "user",
"content": prompt
}
]
)
print(response.selections[0].message.content material)
On this instance, the o1-preview mannequin intelligently plans out the system’s construction and supplies related code snippets, showcasing its problem-solving skills.
Output
Definitely! Creating a list administration system entails a number of elements, together with database fashions,
person interfaces, and enterprise logic for monitoring stock ranges and notifying customers when inventory is low.
Beneath is a plan for the listing construction of your venture, together with code snippets for key elements
utilizing Python and the Flask net framework.
---
## Listing Construction
This is a recommended listing construction in your Flask-based stock administration system:
```
inventory_management/
├── app.py
├── necessities.txt
├── config.py
├── run.py
├── occasion/
│ └── config.py
├── fashions/
│ ├── __init__.py
│ └── product.py
├── routes/
│ ├── __init__.py
│ └── product_routes.py
├── providers/
│ ├── __init__.py
│ └── notification_service.py
├── templates/
│ ├── base.html
│ ├── index.html
│ └── product_detail.html
├── static/
│ ├── css/
│ └── js/
└── db/
└── stock.db
```
- **app.py**: Initializes the Flask app and the database.
- **config.py**: Incorporates configuration variables.
- **run.py**: The entry level to run the applying.
- **fashions/**: Incorporates database fashions.
- **routes/**: Incorporates route handlers for URL endpoints.
- **providers/**: Incorporates service layers like notification providers.
- **templates/**: Incorporates HTML templates for rendering net pages.
- **static/**: Incorporates static information like CSS and JavaScript.
- **db/**: Incorporates the SQLite database file.
---
## Key Elements Code Snippets
### 1. `app.py`: Initialize Flask App and Database
```python
# app.py
from flask import Flask
from flask_sqlalchemy import SQLAlchemy
from config import Config
db = SQLAlchemy()
def create_app():
app = Flask(__name__)
app.config.from_object(Config)
db.init_app(app)
with app.app_context():
from fashions import product
db.create_all()
from routes.product_routes import product_bp
app.register_blueprint(product_bp)
return app
```
### 2. `config.py`: Configuration Settings
```python
# config.py
import os
class Config:
SECRET_KEY = os.environ.get('SECRET_KEY', 'your_secret_key_here')
SQLALCHEMY_DATABASE_URI = 'sqlite:///db/stock.db'
SQLALCHEMY_TRACK_MODIFICATIONS = False
LOW_STOCK_THRESHOLD = 10 # Amount at which to inform for low inventory
```
### 3. `fashions/product.py`: Product Mannequin
```python
# fashions/product.py
from app import db
class Product(db.Mannequin):
id = db.Column(db.Integer, primary_key=True)
title = db.Column(db.String(100), nullable=False)
amount = db.Column(db.Integer, nullable=False, default=0)
location = db.Column(db.String(100), nullable=False)
def __repr__(self):
return f''
```
### 4. `routes/product_routes.py`: Route Handlers
```python
# routes/product_routes.py
from flask import Blueprint, render_template, request, redirect, url_for, flash
from app import db
from fashions.product import Product
from providers.notification_service import check_and_notify_low_stock
product_bp = Blueprint('product_bp', __name__)
@product_bp.route("https://www.analyticsvidhya.com/")
def index():
merchandise = Product.question.all()
return render_template('index.html', merchandise=merchandise)
@product_bp.route('/product/')
def product_detail(product_id):
product = Product.question.get_or_404(product_id)
return render_template('product_detail.html', product=product)
@product_bp.route('/add_product', strategies=['GET', 'POST'])
def add_product():
if request.technique == 'POST':
title = request.type['name']
amount = int(request.type['quantity'])
location = request.type['location']
new_product = Product(title=title, amount=amount, location=location)
db.session.add(new_product)
db.session.commit()
check_and_notify_low_stock(new_product)
flash('Product added efficiently!', 'success')
return redirect(url_for('product_bp.index'))
return render_template('add_product.html')
@product_bp.route('/update_product/', strategies=['GET', 'POST'])
def update_product(product_id):
product = Product.question.get_or_404(product_id)
if request.technique == 'POST':
product.title = request.type['name']
product.amount = int(request.type['quantity'])
product.location = request.type['location']
db.session.commit()
check_and_notify_low_stock(product)
flash('Product up to date efficiently!', 'success')
return redirect(url_for('product_bp.product_detail', product_id=product.id))
return render_template('update_product.html', product=product)
```
### 5. `providers/notification_service.py`: Notification Service
```python
# providers/notification_service.py
from flask import current_app, flash
def check_and_notify_low_stock(product):
if product.amount <= current_app.config['LOW_STOCK_THRESHOLD']:
message = f'Inventory for "{product.title}" is low (Amount: {product.amount})!'
# Implement precise notification logic right here (e.g., ship electronic mail)
flash(message, 'warning')
```
### 6. `templates/index.html`: Show Merchandise
```html
{% extends 'base.html' %}
{% block content material %}
Add New Product
{% for product in merchandise %}
{% endfor %}
Identify
Amount
Location
Actions
{{ product.title }}
{{ product.amount }}
{{ product.location }}
View
Edit
{% endblock %}
```
### 7. `run.py`: Run the Software
```python
# run.py
from app import create_app
app = create_app()
if __name__ == '__main__':
app.run(debug=True)
```
---
## Rationalization of Key Elements
- **Product Mannequin**: Represents the product entity with fields for
`title`, `amount`, and `location`. It interacts with the database utilizing SQLAlchemy.
- **Routes**: Outline endpoints for viewing, including, and updating merchandise.
They deal with HTTP requests and render templates.
- **Notification Service**:
Incorporates logic to examine if the product's amount is beneath the edge outlined within the configuration.
It presently makes use of Flask's `flash` system for notifications,
however you possibly can prolong it to ship emails or push notifications.
- **Templates**: HTML information that construction the presentation layer.
The `index.html` template shows the checklist of merchandise in a desk format.
- **Configuration**: International settings just like the database URI and the low inventory threshold are outlined in `config.py`.
---
## Subsequent Steps
- **Database Migration**: Think about using Flask-Migrate for dealing with database migrations as your fashions evolve.
- **Consumer Authentication**: Implement person login performance if wanted.
- **Enhanced Notifications**: Combine an electronic mail server or a messaging API for sending out low inventory alerts.
- **Error Dealing with**: Add correct error dealing with for robustness.
- **Entrance-Finish Enhancements**: Enhance the person interface with CSS frameworks like Bootstrap or Materialize.
---
## Necessities Set up
To arrange the venture, create a digital atmosphere and set up the required packages:
```bash
python3 -m venv venv
supply venv/bin/activate
pip set up flask flask_sqlalchemy
```
Add these dependencies to your `necessities.txt`:
```
Flask
Flask_SQLAlchemy
```
---
## Operating the Software
After establishing, you possibly can run the applying utilizing:
```bash
python run.py
```
Navigate to `http://localhost:5000` in your net browser to work together with the stock administration system.
---
By following this construction and utilizing these code snippets as a place to begin,
you possibly can develop a useful stock administration system that tracks merchandise, their portions, and places,
and notifies customers when inventory ranges are low.
Scientific Reasoning and Past
The o1-preview mannequin can be wonderful for scientific analysis, the place superior reasoning is required. Right here’s an instance the place the mannequin is requested to discover the potential of CRISPR expertise for treating genetic issues:
Create the Immediate
immediate = """
Clarify how CRISPR expertise can be utilized to deal with genetic issues. What are the
fundamental challenges, and what future developments could be essential to make it broadly
accessible?
"""
Make the API Name
response = shopper.chat.completions.create(
mannequin="o1-preview",
messages=[
{
"role": "user",
"content": prompt
}
]
)
print(response.selections[0].message.content material)
This instance highlights how the o1-preview mannequin can purpose by way of advanced scientific challenges, making it a superb device for researchers and scientists.
Output
**Introduction to CRISPR Know-how**
CRISPR (Clustered Frequently Interspaced Quick Palindromic Repeats) expertise is a groundbreaking gene-editing device that enables scientists to
alter DNA sequences and modify gene perform with unprecedented precision and effectivity.
Derived from a pure protection mechanism present in micro organism and archaea, CRISPR-Cas methods defend these microorganisms from viral infections.
Essentially the most generally used system in gene modifying is CRISPR-Cas9, the place the Cas9 enzyme acts as molecular scissors to chop DNA at a particular location
guided by a customizable RNA sequence.
**Utilizing CRISPR to Deal with Genetic Problems**
Genetic issues are sometimes brought on by mutations or alterations in a person's DNA that disrupt regular gene perform. CRISPR expertise can
probably right these mutations on the genetic degree, providing the prospect of curing illnesses fairly than simply managing signs.
The final steps concerned in utilizing CRISPR for treating genetic issues embrace:
1. **Identification of the Goal Gene:** Figuring out the precise genetic mutation liable for the dysfunction.
2. **Designing the Information RNA (gRNA):** Crafting a sequence of RNA that matches the DNA sequence on the mutation website.
3. **Supply into Goal Cells:** Introducing the CRISPR-Cas9 elements into the affected person's cells, both ex vivo (outdoors the physique) or in vivo (contained in the physique).
4. **Gene Modifying Course of:** As soon as contained in the cells, the Cas9 enzyme, guided by the gRNA, binds to the goal DNA sequence and introduces a minimize.
The cell's pure restore mechanisms then take over to repair the minimize, ideally correcting the mutation.
5. **Restoration of Regular Perform:** If profitable, the gene is corrected, and regular protein manufacturing and mobile capabilities are restored,
assuaging or eliminating illness signs.
**Essential Challenges in Utilizing CRISPR for Treating Genetic Problems**
Whereas CRISPR holds immense potential, a number of important challenges should be addressed earlier than it may change into a routine therapy for genetic issues:
1. **Supply to Goal Cells and Tissues:**
- **Environment friendly Supply Strategies:** Getting the CRISPR elements into the precise cells within the physique is a significant hurdle. Supply strategies should be environment friendly,
particular to the goal cells, and secure.
- **Viral Vectors Limitations:** Frequent supply strategies use viral vectors (like adenoviruses or adeno-associated viruses),
which may provoke immune responses or have measurement limitations for the genetic payload.
- **Non-Viral Supply Methods:** Options like lipid nanoparticles are being explored, however optimizing their effectivity and specificity stays difficult.
2. **Off-Goal Results and Unintended Mutations:**
- **Precision Considerations:** Cas9 may minimize at unintended websites within the genome, resulting in off-target results that would trigger dangerous mutations or disrupt different genes.
- **Genome Integrity:** Making certain that gene modifying doesn't inadvertently enhance the chance of most cancers or different genetic illnesses is essential.
3. **Immune Responses:**
- **Immunogenicity of CRISPR Elements:** The Cas9 protein is of bacterial origin and could also be acknowledged as international by the human immune system,
resulting in immune reactions that may scale back therapy efficacy or trigger opposed results.
4. **Moral and Regulatory Points:**
- **Germline Modifying Considerations:** Modifying genes in germline cells (sperm, eggs, or embryos) raises moral questions
as a result of adjustments are heritable and may have an effect on future generations.
- **Regulatory Approval:** Strong regulatory frameworks are wanted to supervise scientific functions, guarantee affected person security, and deal with bioethical concerns.
5. **Lengthy-Time period Results and Security:**
- **Sturdiness of Gene Correction:** It's needed to know how lengthy the consequences of gene modifying final and whether or not repeated remedies are wanted.
- **Monitoring for Adversarial Outcomes:** Lengthy-term research are required to observe for delayed opposed results, corresponding to tumor improvement.
6. **Technical Limitations:**
- **Gene Supply Dimension Constraints:** Some genes are too massive to be effectively delivered utilizing present vectors.
- **Management Over Gene Restore Mechanisms:** The cell's pure DNA restore pathways could not all the time end result within the
desired gene correction after Cas9-induced cuts.
**Future Developments Wanted for Widespread Availability**
To beat these challenges and make CRISPR-based therapies broadly accessible, a number of developments and developments are needed:
1. **Enhanced Supply Methods:**
- **Focused Supply Mechanisms:** Creating secure, environment friendly, and exact strategies to ship CRISPR
elements particularly to affected cells or tissues.
- **Non-Viral Vectors:** Advancing non-viral supply strategies like nanoparticles, engineered exosomes,
or bodily strategies (e.g., electroporation) to cut back immunogenicity and enhance payload capability.
2. **Bettering Specificity and Decreasing Off-Goal Results:**
- **Excessive-Constancy Cas9 Variants:** Engineering Cas9 enzymes with greater specificity to reduce unintended cuts.
- **Various Nucleases:** Exploring different enzymes (e.g., Cas12a) or base editors that may make
exact adjustments with out introducing double-strand breaks.
3. **Security Enhancements:**
- **Transient Expression Methods:** Designing CRISPR elements which are lively solely briefly to
scale back the window for off-target exercise.
- **Immunomodulation Methods:** Creating approaches to mitigate immune responses in opposition to CRISPR elements.
4. **Moral Pointers and Regulatory Frameworks:**
- **Worldwide Consensus:** Establishing world moral requirements and rules for permissible
makes use of of gene modifying, notably regarding germline modifications.
- **Public Engagement:** Educating the general public and stakeholders to construct belief and knowledgeable consent concerning gene-editing therapies.
5. **Medical Trials and Lengthy-Time period Research:**
- **Strong Medical Knowledge:** Conducting in depth scientific trials to show security and efficacy
throughout various populations and genetic issues.
- **Monitoring and Reporting Methods:** Implementing long-term monitoring for sufferers who
endure CRISPR-based remedies to trace outcomes and negative effects.
6. **Scalability and Price Discount:**
- **Manufacturing Advances:** Bettering the manufacturing processes for CRISPR elements to make therapies extra reasonably priced.
- **Healthcare Infrastructure:** Enhancing healthcare methods to assist the supply and follow-up care required for gene-editing remedies.
7. **Personalised Drugs Integration:**
- **Genetic Screening and Diagnostics:** Incorporating complete genetic
testing to determine sufferers who can profit from CRISPR therapies.
- **Personalized Remedies:** Creating protocols for tailoring CRISPR therapies to particular person genetic profiles.
8. **Regulatory Approval Pathways:**
- **Streamlined Processes:** Working with regulatory our bodies to ascertain clear pathways
for the approval of gene-editing therapies whereas making certain affected person security.
- **Collaborative Efforts:** Encouraging collaboration between academia,
business, and regulatory businesses to speed up improvement and approval.
**Conclusion**
CRISPR expertise holds transformative potential for treating genetic issues by offering a
means to right disease-causing mutations at their supply.
Nevertheless, important scientific, technical, moral, and regulatory challenges should
be addressed to comprehend this potential totally. Developments in supply strategies, specificity, security,
moral tips, and regulatory frameworks are essential.
With continued analysis, collaboration, and accountable innovation, CRISPR-based therapies could
change into a broadly accessible actuality, providing hope to people affected by genetic illnesses.
Additionally Learn: 3 Palms-On Experiments with OpenAI’s o1 You Have to See
Conclusion
The OpenAI o1 fashions are a breakthrough in reasoning-based AI, able to dealing with advanced programming, mathematical, and scientific duties. Whether or not you’re engaged on algorithms, refactoring code, or planning functions, the o1 API affords a robust toolset for creating options that require deep thought and superior logic.
Whereas nonetheless in beta, these fashions promise much more capabilities sooner or later, corresponding to multimodality and gear utilization. In case your initiatives demand superior reasoning and don’t require speedy response instances, the o1 fashions might be your go-to choice.
This model makes use of totally different prompts to show the flexibleness and reasoning skills of the OpenAI o1 fashions throughout varied duties.
Keep tuned to Analytics Vidhya weblog to know extra in regards to the makes use of of o1!
Regularly Requested Questions
A. OpenAI o1 fashions are superior AI fashions particularly designed to excel in advanced reasoning duties, together with math, science, and coding. They’re constructed to interact in deeper considering earlier than producing responses, permitting them to deal with intricate issues extra successfully.
A. The o1-preview is a full-featured mannequin able to tackling advanced duties with enhanced reasoning skills, making it appropriate for a variety of functions. However, o1-mini is a sooner, extra cost-efficient model optimized for coding and reasoning duties, working at 80% of the price of o1-preview.
A. The o1 fashions are acknowledged for his or her distinctive efficiency in coding, fixing math issues, and understanding scientific ideas. They’ve demonstrated superior outcomes in comparison with earlier fashions in standardized checks just like the AIME math examination and the GPQA-diamond for scientific reasoning.
A. ChatGPT Plus and Workforce customers have entry to the o1 fashions at the moment with sure message limits. ChatGPT Enterprise and Edu customers could have entry subsequent week. Builders may use the fashions by way of API at utilization tier 5.
A. The o1 fashions are perfect for researchers and scientists tackling advanced duties corresponding to gene sequencing and superior scientific computations. Builders can leverage these fashions for highly effective coding and workflow optimization. College students and educators can use them to discover difficult math and science issues.