Frida, a preferred open-source challenge, is a dynamic instrumentation toolkit that allows builders, safety researchers and reverse engineers to inject customized scripts into operating processes. It permits customers to carry out quite a lot of duties corresponding to perform hooking, API name tracing and runtime manipulation, making it a flexible software for debugging, reverse engineering and cellular utility safety testing. Frida helps a number of platforms and launched iOS 17 help in model 16.3.0. What follows is a behind-the-scenes recounting of how we achieved iOS 17 help (and past) in Frida.
Writing software program that observes the conduct of different software program is enjoyable. Having spent a few many years doing this, creating the Frida toolkit within the course of, there’s one factor that is still fixed: every new working system launch could require vital effort to help.
This occurs for one in all two causes. The primary is that OSes lack a number of of the general public APIs that we’d like, so we find yourself counting on undocumented internals, and people internals have a tendency to vary. The second is that interfacing with a cellular system entails numerous communication protocols, additionally sometimes undocumented, and people additionally have a tendency to vary.
CoreDevice
Beginning with iOS 17, Apple moved to a brand new means of speaking with companies on the iDevice aspect, together with its Developer Disk Picture (DDI) companies. Thisnew means of doing issues unifies how Apple software program talks to different Apple gadgets, and is called CoreDevice. It appears to have originated again when Apple launched the T2 coprocessor. The Cisco DUO workforce revealed some glorious analysis on this again in 2019.
Like with the T2, the brand new stack used for iOS 17 additionally makes use of RemoteXPC for speaking to companies. Issues are a bit extra complicated although, as a result of not like the T2, cellular gadgets don’t have a persistent connection to macOS, and now have the notion of pairing. Fortunately for us, @doronz88 had already completed an incredible job reversing and documenting most of what we wanted to implement the brand new protocols, in order that saved us loads of time.
Even so, it was a large enterprise, however tons of enjoyable — @hsorbo and I had a blast pair-programming on it. Fairly a couple of shifting components are concerned, so let’s take a fast stroll via them.
It was a large enterprise, however tons of enjoyable.
With an iDevice plugged in, it exposes a USB CDC-NCM community interface, referred to as the “personal” interface. It additionally exposes an interface used for tethering, identical to it did previously — besides again then it was utilizing a proprietary Apple protocol as a substitute of CDC-NCM.
That is the place we hit the primary challenges making an attempt to speak to it from a Linux host. First off, the iOS system wants a USB vendor request to mode-switch it into exposing the brand new interfaces. macOS does this routinely, and we’ve enhanced Frida to additionally do that if wanted. That is additionally completed by usbmuxd if utilizing a considerably current git snapshot, however on condition that the brand new CoreDevice help makes usbmux redundant, we figured it could be good to not require or not it’s put in and up-to-date.
The subsequent problem was that the Linux kernel’s CDC-NCM driver did not bind to the system. After a little bit of debugging we found that it was because of the personal community interface missing a standing endpoint. The standing endpoint is how the driving force will get notified about whether or not a community cable is plugged in. Apple’s tethering community interface has such an endpoint, and it is sensible there — if tethering is disabled it’s simply as if the cable is unplugged. However the personal interface is all the time there, so understandably Apple selected to not embrace a standing endpoint for it.
We rapidly developed a kernel driver patch to elevate the requirement for a standing endpoint, and this acquired it working. Later we realized that we should always nonetheless require a standing endpoint for the tethering interface, so we ended up refining our patch a bit additional. We submitted our refined patch, which is now upstream, and might be a part of Linux v6.11 as soon as that’s launched.
Till then nonetheless, and for customers on OSes with no appropriate NCM driver, we carried out a minimal user-mode driver that Frida now makes use of when it detects that the kernel doesn’t present one. We leveraged lwIP to additionally do Ethernet and IPv6 fully in consumer house. The result’s that Frida can help CoreDevice on any platform supported by libusb.
Anyway, with the community interface up, the host aspect makes use of mDNS to find the IPv6 handle the place a RemoteServiceDiscovery (RSD) service is listening. The host connects to it and speaks HTTP/2 with RemoteXPC messages going backwards and forwards. This explicit service, RSD, tells the host which companies can be found on the personal interface, the port numbers they’re listening on, and particulars just like the protocol every makes use of to speak.
Then, figuring out which companies are listening on which ports, the host seems up the Tunnel service. This service lets the host set up a tunnel to the system, appearing as a VPN to permit it to speak with the companies inside that tunnel. Since establishing such a tunnel requires a pairing relationship between the host and the system, it signifies that the companies contained in the tunnel enable the host to do much more issues than the companies exterior the tunnel.
The bottom protocol is similar as with RSD, and after some backwards and forwards involving a pairing parameters binary blob and a few cryptography, a pairing relationship is both created or verified. At this level the 2 endpoints are utilizing encrypted communications, and the host asks the Tunnel service to arrange a tunnel listener.
Now, assuming the host requested for the default transport, QUIC, the host goes forward and connects to it. We must always be aware that the Tunnel service additionally helps plain TCP. Presumably that’s there for older macOS variations that don’t include a QUIC stack. One other factor value mentioning is that the Tunnel service supplies the host with a keypair, so it makes use of that as a part of the connection setup.
As soon as linked, the system sends the host some information throughout a dependable stream. The information begins with an 8 byte magic, “CDTunnel”, adopted by a big-endian uint16 that specifies the scale of the payload following it. The payload is JSON, and tells the host which IPv6 handle the host’s endpoint contained in the tunnel has, together with the netmask and MTU. It additionally tells the host its personal IPv6 handle contained in the tunnel, and the port that the RSD service is listening on.
The host then units up a TUN system configured because it was simply advised, and begins feeding it unreliable datagrams as they’re obtained from the QUIC connection. And for information within the different course, each time there’s a brand new packet from the TUN system, the host feeds that into the QUIC connection.
So at this level the host connects to the RSD endpoint contained in the tunnel, and from there it may well entry the entire companies that the system has to supply. The great thing about this new method is that shoppers speaking with device-side companies don’t have to fret about crypto, nor present proof of a pairing relationship. They’ll merely make plaintext TCP connections on the tunnel interface, and QUIC handles the remainder transparently.
What’s even cooler is that the host can set up tunnels throughout each USB and WiFi/a community interface, and due to QUIC’s native help for multipath, the system can seamlessly transfer between wired and wi-fi with out disrupting connections to companies contained in the tunnel.
So, as soon as we acquired all of this carried out we had been feeling excited and optimistic. The one half left was to do the platform integrations. And uhh yeah, that’s the place issues acquired loads more durable. The half that acquired us caught for some time was on macOS, the place we realized we needed to piggyback on Apple’s present tunnel. We found that the Tunnel service would refuse to speak to us when there’s already a tunnel open, so we couldn’t merely open up a tunnel subsequent to Apple’s.
Whereas we may ask the consumer to ship SIGSTOP to isolated, permitting us to arrange our personal tunnel, it wouldn’t be an incredible consumer expertise. Particularly since any Apple software program wanting to speak to the system then wouldn’t have the ability to, making Xcode, Console, and so on. loads much less helpful.
It didn’t take us lengthy to seek out personal APIs that may enable us to find out the device-side handle inside Apple’s tunnel, and likewise create a so-called “assertion” in order that the tunnel is saved open for so long as we’d like it. However the half we couldn’t determine was how you can uncover the device-side RSD port contained in the tunnel.
We knew that remotepairingd, operating because the native consumer, is aware of the RSD port, however we couldn’t discover a solution to get it to inform us what it’s. After numerous brainstorming we may solely consider impractical options:
- Port-scan the device-side handle: Doubtlessly gradual, and quicker implementations would require root for uncooked socket entry.
- Scan remotepairingd’s handle house for the device-side tunnel handle, and find the port saved close to it: Wouldn’t work with SIP enabled.
- Depend on a device-side frida-server to determine issues out for us: This wouldn’t work on jailed iOS, and can be complicated and probably fragile.
- Seize it from the syslog: Might take some time or require a guide consumer motion, and forcing a reconnection by killing a system daemon would lead to disruption.
- Surrender on utilizing the tunnel, and transfer to a better stage abstraction, e.g. use MobileDevice.framework each time we have to open a service: Would require us to own entitlements. Precisely which rely on the actual service.For instance if we’d need to discuss to com.apple.coredevice.appservice, we’d want the com.apple.personal.CoreDevice.canInstallCustomerContent entitlement. However, making an attempt to provide ourselves a com.apple.personal.* entitlement simply wouldn’t fly, because the system would kill us as a result of solely Apple-signed packages can use such entitlements.
This was the place we determined to take a break and concentrate on different issues for some time, till we lastly discovered a means: The isolated course of has a connection to the RSD service contained in the tunnel. We lastly arrived at a easy resolution, utilizing the identical API that Apple’s netstat is utilizing:
The non-macOS aspect of the story was loads simpler although, as there we’re in management and may arrange a tunnel ourselves. There was one problem nonetheless: We didn’t need to require elevated privileges to have the ability to create a tun system. The answer we got here up with was to make use of lwIP to do IPv6 in user-mode. As we had already designed our different constructing blocks to work with GLib.IOStream, decoupled from sockets and networking, all we needed to do was implement an IOStream that makes use of lwIP to do the heavy lifting. Datagrams from the QUIC connection get fed into an lwIP community interface, and packets emitted by that community interface are fed into the QUIC connection as datagrams.
As soon as we acquired all of that working, we additionally went the additional mile and carried out help for community connectivity, so the USB cable could be unplugged. The pairing step nonetheless requires it although, as a result of Apple’s iOS/iPadOS coverage is to solely enable pairing throughout the NCM interface. It’s value mentioning that tvOS permits it, however we didn’t but get an opportunity to check that half.
So with all of that working, the one factor left was to boost frida-server and frida-gadget in order that they pay attention on the personal interfaces as they seem. That is the place we leveraged SystemConfiguration.framework to be notified because the community interfaces come and go.
Jailed iOS 17
With the brand new CoreDevice infrastructure in place, we additionally went forward and restored help for jailed instrumentation on iOS 17. This implies we are able to as soon as once more spawn (debuggable) apps on newest iOS, which is 17.5.1 on the time of writing. We additionally fastened the difficulty the place connect() with out spawn() wouldn’t work on current iOS variations.
iOS 18 betas
We additionally went forward and explored the most recent betas, and tailored Frida to the brand new modifications in Apple’s dynamic linker. In order of the most recent Frida 16.4, there’s now additionally help for iOS 18 and macOS Sequoia. Thrilling instances forward!
System.open_service() and the Service API
On condition that Frida wants to talk fairly a couple of protocols to work together with Apple’s device-side companies, and our merchandise additionally want different such companies, this presents a problem. We may communicate these protocols ourselves, e.g. after utilizing the System.open_channel() API to open an IOStream to a particular service. However this implies we’d need to duplicate the hassle of implementing and sustaining the protocol stacks, and for protocols corresponding to DTX we’d be losing time establishing a connection that Frida already established for its personal wants.
One attainable resolution can be to make these service shoppers public API and expose them in Frida’s language bindings. We’d additionally need to implement shoppers for the entire Apple companies that our merchandise need to discuss to. That’s fairly a couple of, and would outcome within the Frida API changing into large. It might additionally make Frida a kitchen sink of kinds, and that’s clearly not a course we need to be heading in.
After desirous about this for some time, it occurred to me that we may present a generic abstraction that lets the appliance discuss to any service that they need. So @hsorbo and I crammed up our espresso cups and set to work. We’re fairly proud of the way it turned out.
Right here’s how straightforward it’s to speak to a RemoteXPC service from Python:
And the identical instance, from Node.js:
Which leads to:
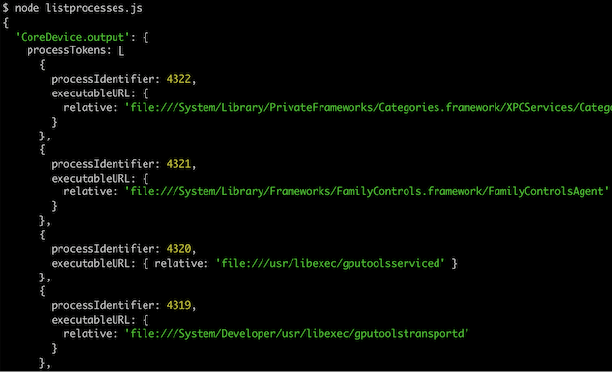
So now that we’ve appeared on the new open_service() API getting used from Python and Node.js, we should always in all probability point out that it’s (nearly) simply as straightforward to make use of this API from C:
(Error-handling and cleanup omitted for brevity.)
The string handed into open_service() is the handle the place the service could be reached, which begins with a protocol identifier adopted by colon and the service identify. This returns an implementation of the Service interface, which seems like this:
(Synchronous strategies omitted for brevity.)
Right here, request() is what you name with a Variant, which could be “something”, a dictionary, array, string, and so on. It’s as much as the precise protocol what is predicted. Our language bindings handle turning a local worth, for instance a dict in case of Python, right into a Variant. As soon as request() returns, it offers you a Variant with the response. That is then became a local worth, e.g. a Python dict.
For protocols that help notifications, the message sign is emitted each time a notification is obtained. Since Frida’s APIs additionally provide synchronous variations of all strategies, permitting them to be known as from an arbitrary thread, this presents a problem: If a message is emitted as quickly as you open a particular service, you is likely to be too late in registering the handler. That is the place activate() comes into play. The service object begins out within the inactive state, permitting sign handlers to be registered. Then, when you’re prepared for occasions, you’ll be able to both name activate(), or make a request(), which strikes the service object into lively state.
Then, later, to close issues down, cancel() could also be known as. The shut sign is helpful to know when a Service is now not obtainable, e.g. as a result of the system was unplugged otherwise you despatched it an invalid message inflicting it to shut the connection.
It’s additionally straightforward to speak to DTX companies, which is RemoteXPC’s predecessor, nonetheless utilized by many DDI companies.
For instance, to seize a screenshot:
However there’s extra. We additionally help speaking to old-style plist companies, the place you ship a plist because the request, and obtain a number of plist responses:
As you may need already guessed, this instance places the linked iDevice to sleep.
iOS 17 and past
NowSecure contributes considerably to open-source neighborhood initiatives corresponding to Frida and Radare and trade requirements from the OWASP Cell Utility Safety Challenge (MAS). NowSecure Platform automated cellular utility safety testing software program makes use of Frida to carry out quick, deep safety and privateness evaluation of cellular apps at scale. Frida help for iOS 17 powers NowSecure Platform danger assessments of the most recent iOS cellular apps. Contact us for a demo of NowSecure Platform.