Knowledge engineering groups are steadily tasked with constructing bespoke ingestion options for myriad customized, proprietary, or industry-specific knowledge sources. Many groups discover that this work of constructing ingestion options is cumbersome and time-consuming. Recognizing these challenges, we’ve got interviewed quite a few corporations throughout completely different industries to higher perceive their numerous knowledge integration wants. This complete suggestions led us to the event of the Python Knowledge Supply API for Apache Spark™.
One of many clients we’ve got labored carefully with is Shell. Tools failures within the power sector can have important penalties, impacting security, the setting, and operational stability. At Shell, minimizing these dangers is a precedence, and a technique they do that is by specializing in the dependable operation of apparatus.
Shell owns an enormous array of capital property and tools valued at over $180 billion. To handle the huge quantities of information that Shell’s operations generate, they depend on superior instruments that improve productiveness and permit their knowledge groups to work seamlessly throughout numerous initiatives. The Databricks Knowledge Intelligence Platform performs an important position by democratizing knowledge entry and fostering collaboration amongst Shell’s analysts, engineers, and scientists. Nevertheless, integrating IoT knowledge posed challenges for some use instances.
Utilizing our work with Shell for instance, this weblog will discover how this new API addresses earlier challenges and supply instance code for example its software.
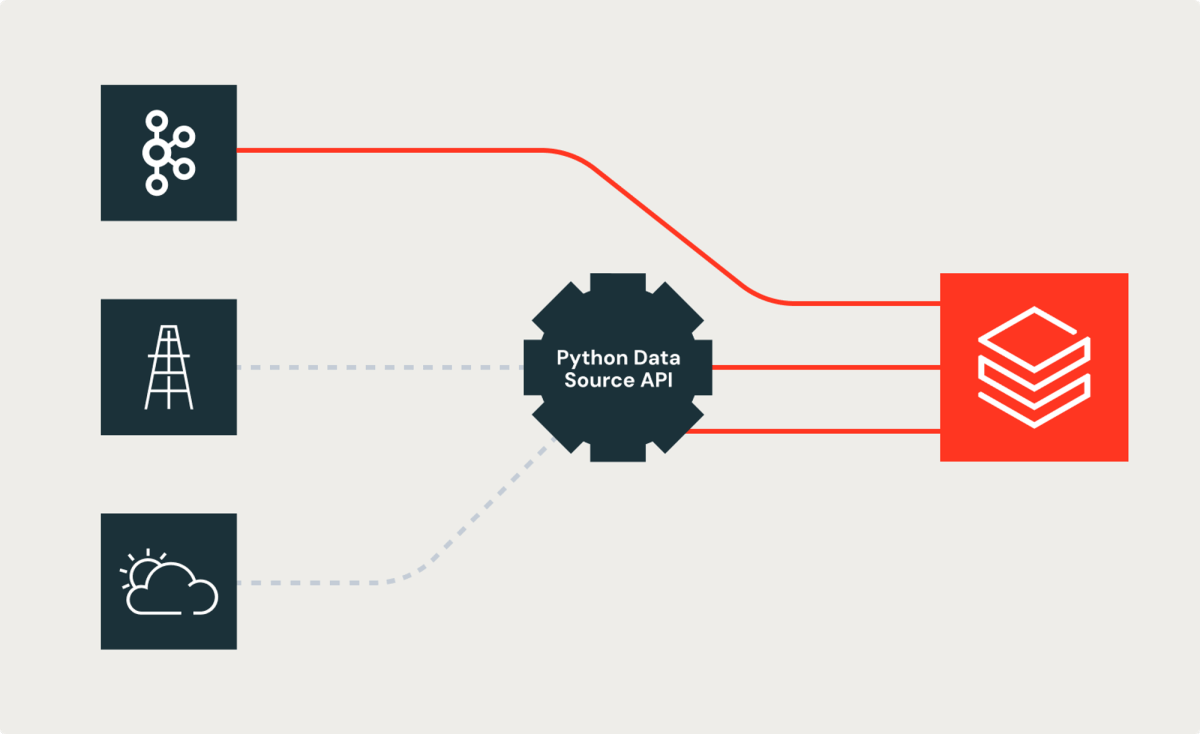
The problem
First, let’s take a look at the problem that Shell’s knowledge engineers skilled. Though many knowledge sources of their knowledge pipelines use built-in Spark sources (e.g., Kafka), some depend on REST APIs, SDKs, or different mechanisms to show knowledge to shoppers. Shell’s knowledge engineers struggled with this truth. They ended up with bespoke options to affix knowledge from built-in Spark sources with knowledge from these sources. This problem burned knowledge engineers’ time and power. As typically seen in massive organizations, such bespoke implementations introduce inconsistencies in implementations and outcomes. Bryce Bartmann, Shell’s Chief Digital Expertise Advisor, needed simplicity, telling us, “We write quite a lot of cool REST APIs, together with for streaming use instances, and would love to simply use them as a knowledge supply in Databricks as an alternative of writing all of the plumbing code ourselves.”
“We write quite a lot of cool REST APIs, together with for streaming use instances, and would love to simply use them as a knowledge supply in Databricks as an alternative of writing all of the plumbing code ourselves.”
– Bryce Bartmann, Chief Digital Expertise Advisor, Shell
The answer
The brand new Python customized knowledge supply API alleviates the ache by permitting the issue to be approached utilizing object-oriented ideas. The brand new API supplies summary lessons that enable customized code, equivalent to REST API-based lookups, to be encapsulated and surfaced as one other Spark supply or sink.
Knowledge engineers need simplicity and composability. For example, think about you’re a knowledge engineer and need to ingest climate knowledge in your streaming pipeline. Ideally, you want to write code that appears like this:
df = spark.readStream.format("climate")
That code appears easy, and it’s straightforward to make use of for knowledge engineers as a result of they’re already acquainted with the DataFrame API. Beforehand, a typical strategy to accessing a REST API in a Spark job was to make use of a PandasUDF. This text reveals how sophisticated it may be to put in writing reusable code able to sinking knowledge to a REST API utilizing a Pandas UDF. The brand new API, alternatively, simplifies and standardizes how Spark jobs – streaming or batch, sink or supply – work with non-native sources and sinks.
Subsequent, let’s look at a real-world instance and present how the brand new API permits us to create a brand new knowledge supply (“climate” on this instance). The brand new API supplies capabilities for sources, sinks, batch, and streaming and the instance beneath focuses on utilizing the brand new streaming API to implement a brand new “climate” supply.
Utilizing the Python Knowledge Supply API – a real-world situation
Think about you’re a knowledge engineer tasked with constructing a knowledge pipeline for a predictive upkeep use case that requires strain knowledge from wellhead tools. Let’s assume the wellhead’s temperature and strain metrics move by Kafka from the IoT sensors. We all know Structured Streaming has native help for processing knowledge from Kafka. Thus far, so good. Nevertheless, the enterprise necessities current a problem: the identical knowledge pipeline should additionally seize the climate knowledge associated to the wellhead web site, and this knowledge simply so occurs to not be streaming by Kafka and is as an alternative accessible through a REST API. The enterprise stakeholders and knowledge scientists know that climate impacts the lifespan and effectivity of apparatus, and people elements influence tools upkeep schedules.
Begin easy
The brand new API supplies a easy choice appropriate for a lot of use instances: the SimpleDataSourceStreamReader
API. The SimpleDataSourceStreamReader
API is acceptable when the information supply has low throughput and doesn’t require partitioning. We are going to use it on this instance as a result of we solely want climate knowledge readings for a restricted variety of wellhead websites, and the frequency of climate readings is low.
Let us take a look at a easy instance that makes use of the SimpleDataSourceStreamReader
API.
We are going to clarify a extra sophisticated strategy later. The opposite, extra advanced strategy is right when constructing a partition-aware Python Knowledge Supply. For now, we can’t fear about what meaning. As an alternative, we are going to present an instance that makes use of the straightforward API.
Code instance
The code instance beneath assumes that the “easy” API is adequate. The __init__
methodology is important as a result of that’s how the reader class (WeatherSimpleStreamReader
beneath) understands the wellhead websites that we have to monitor. The category makes use of a “places” choice to determine places to emit climate data.
import ast
import requests
import json
from pyspark.sql.datasource import SimpleDataSourceStreamReader
from pyspark.sql.varieties import StructType
class WeatherSimpleStreamReader(SimpleDataSourceStreamReader):
def initialOffset(self):
"""
Returns the preliminary offset for studying, which serves because the beginning
level for the streaming knowledge supply.
The preliminary offset is returned as a dictionary the place every secret's a
distinctive identifier for a selected (latitude, longitude) pair, and every
worth is a timestamp string (in ISO 8601 format) representing the purpose
in time from which knowledge ought to begin being learn.
Instance:
For places [(37.7749, -122.4194), (40.7128, -74.0060)], the
offset may appear to be:
{
"offset_37.7749_-122.4194": "2024-09-01T00:00:00Z",
"offset_40.7128_-74.0060": "2024-09-01T00:00:00Z"
}
"""
return {f"offset_{lat}_{lengthy}": "2024-09-01T00:00:00Z" for (lat, lengthy)
in self.places}
@staticmethod
def _parse_locations(locations_str: str):
"""Converts string illustration of checklist of tuples to precise checklist
of tuples."""
return [tuple(map(float, x)) for x in ast.literal_eval(locations_str)]
def __init__(self, schema: StructType, choices: dict):
"""Initialize with schema and choices."""
tremendous().__init__()
self.schema = schema
self.places = self._parse_locations(choices.get("places", "[]"))
self.api_key = choices.get("apikey", "")
self.present = 0
self.frequency = choices.get("frequency", "minutely")
self.session = requests.Session() # Use a session for connection pooling
def learn(self, begin: dict):
"""Reads knowledge ranging from the given offset."""
knowledge = []
new_offset = {}
for lat, lengthy in self.places:
start_ts = begin[f"offset_{lat}_{long}"]
climate = self._fetch_weather(lat, lengthy, self.api_key, self.session)[self.frequency]
for entry in climate:
# Begin time is unique and finish time is inclusive.
if entry["time"] > start_ts:
knowledge.append((lat, lengthy, json.dumps(entry["values"]),
entry["time"]))
new_offset.replace({f"offset_{lat}_{lengthy}": climate[-1]["time"]})
return (knowledge, new_offset)
@staticmethod
def _fetch_weather(lat: float, lengthy: float, api_key: str, session):
"""Fetches climate knowledge for the given latitude and longitude utilizing a REST API."""
url = f"https://api.tomorrow.io/v4/climate/forecast?location={lat},{lengthy}&apikey={api_key}"
response = session.get(url)
response.raise_for_status()
return response.json()["timelines"]
Now that we’ve got outlined the straightforward reader class, we have to wire it into an implementation of the DataSource
summary class.
from pyspark.sql.datasource import DataSource
from pyspark.sql.varieties import StructType, StructField, DoubleType, StringType
class WeatherDataSource(DataSource):
"""
A customized PySpark knowledge supply for fetching climate knowledge from tomorrow.io for
given places (latitude, longitude).
Choices
-------
- places: specify an inventory of (latitude, longitude) tuples.
- apikey: specify the API key for the climate service (tomorrow.io).
- frequency: specify the frequency of the information ("minutely", "hourly",
"day by day"). Default is "minutely".
"""
@classmethod
def title(cls):
"""Returns the title of the information supply."""
return "climate"
def __init__(self, choices):
"""Initialize with choices offered."""
self.choices = choices
self.frequency = choices.get("frequency", "minutely")
if self.frequency not in ["minutely", "hourly", "daily"]:
elevate ValueError(f"Unsupported frequency: {self.frequency}")
def schema(self):
"""Defines the output schema of the information supply."""
return StructType([
StructField("latitude", DoubleType(), True),
StructField("longitude", DoubleType(), True),
StructField("weather", StringType(), True),
StructField("timestamp", StringType(), True),
])
def simpleStreamReader(self, schema: StructType):
"""Returns an occasion of the reader for this knowledge supply."""
return WeatherSimpleStreamReader(schema, self.choices)
Now that we’ve got outlined the DataSource and wired in an implementation of the streaming reader, we have to register the DataSource with the Spark session.
spark.dataSource.register(WeatherDataSource)
Meaning the climate knowledge supply is a brand new streaming supply with the acquainted DataFrame operations that knowledge engineers are snug utilizing. This level is price stressing as a result of these customized knowledge sources profit the broader crew. With a extra object-oriented strategy, the broader crew ought to profit from this knowledge supply ought to they want climate knowledge as a part of their use case. Thus, the information engineers could need to extract the customized knowledge sources right into a Python wheel library for reuse in different pipelines.
Under, we see how straightforward it’s for the information engineer to leverage the customized stream.
websites = """[
(60.3933, 5.8341), # Snorre Oil Field, Norway
(58.757, 2.198), # Schiehallion, UK
(58.871, 4.862), # Clair field, UK
(57.645, 3.164), # Elgin-Franklin, UK
(54.932, -5.498), # Sean field, UK
(-14.849, 12.395), # Angola offshore
(1.639, 100.468), # Malampaya, Philippines
(-27.0454, 152.1213), # Australia offshore
(38.1, -119.8), # California offshore
(52.784, 1.698) # Leman, North Sea
]"""
show(
spark.readStream.format("climate")
.choice("places", websites)
.choice("apikey", "tomorrow_io_api_key")
.load()
)
Instance outcomes:
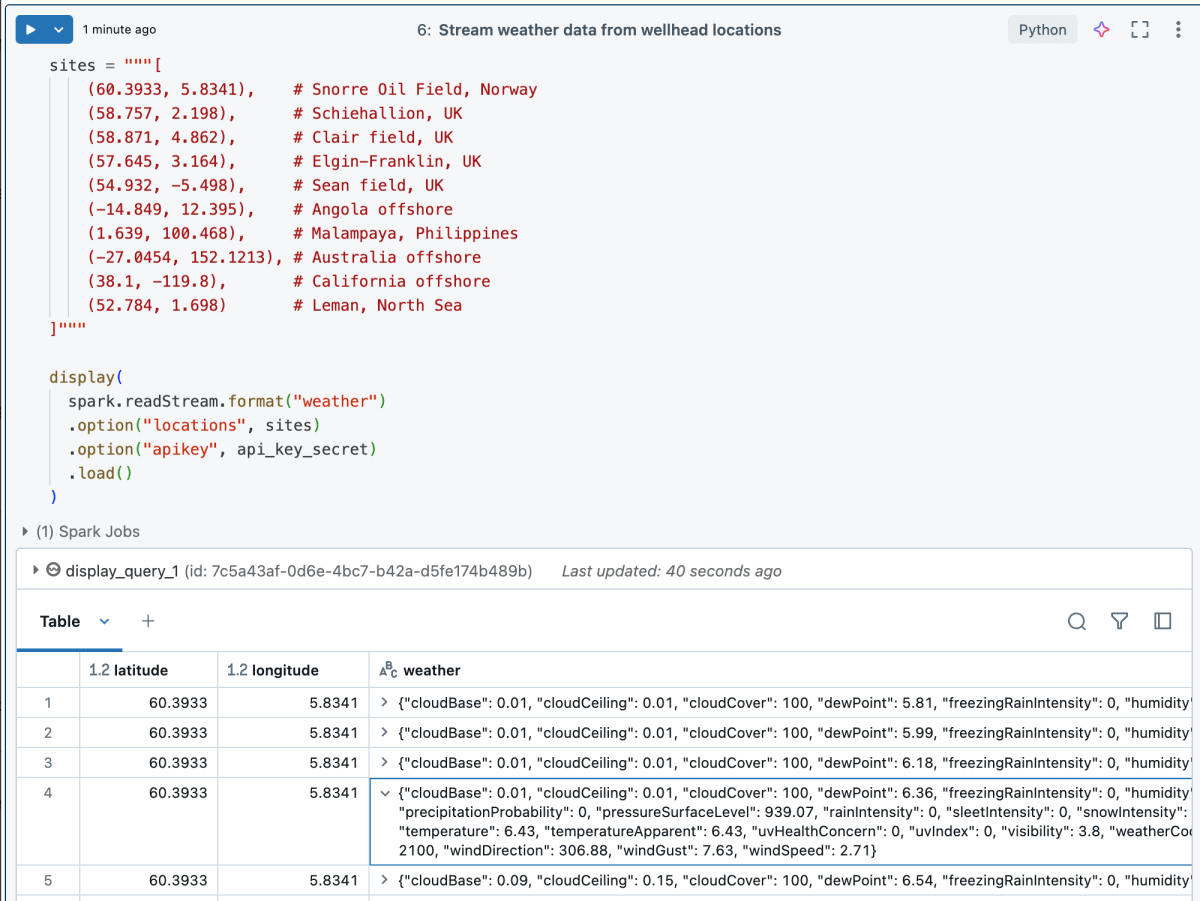
Different concerns
When to make use of the partition-aware API
Now that we’ve got walked by the Python Knowledge Supply’s “easy” API, we are going to clarify an choice for partition consciousness. Partition-aware knowledge sources assist you to parallelize the information era. In our instance, a partition-aware knowledge supply implementation would lead to employee duties dividing the places throughout a number of duties in order that the REST API calls can fan out throughout employees and the cluster. Once more, our instance doesn’t embody this sophistication as a result of the anticipated knowledge quantity is low.
Batch vs. Stream APIs
Relying on the use case and whether or not you want the API to generate the supply stream or sink the information, you need to give attention to implementing completely different strategies. In our instance, we don’t fear about sinking knowledge. We additionally ought to have included the batch reader implementation. Nevertheless, you’ll be able to give attention to implementing the mandatory lessons in your particular use case.
supply | sink | |
---|---|---|
batch | reader() | author() |
streaming | streamReader() or simpleStreamReader() | streamWriter() |
When to make use of the Author APIs
This text has targeted on the Reader APIs used within the readStream
. The author APIs enable comparable arbitrary logic on the output facet of the information pipeline. For instance, let’s assume that the operations managers on the wellhead need the information pipeline to name an API on the wellhead web site that reveals a crimson/yellow/inexperienced tools standing that leverages the pipeline’s logic. The Author API would enable knowledge engineers the identical alternative to encapsulate the logic and expose a knowledge sink that may function like acquainted writeStream
codecs.
Conclusion
“Simplicity is the final word sophistication.” – Leonardo da Vinci
As architects and knowledge engineers, we now have a chance to simplify batch and streaming workloads utilizing the PySpark customized knowledge supply API. As you discover alternatives for brand new knowledge sources that may profit your knowledge groups, contemplate separating the information sources for reuse throughout the enterprise, for instance, by the usage of a Python wheel.
The Python Knowledge Supply API is strictly what we would have liked. It supplies a chance for our knowledge engineers to modularize code mandatory for interacting with our REST APIs and SDKs. The truth that we will now construct, take a look at, and floor reusable Spark knowledge sources throughout the org will assist our groups transfer quicker and have extra confidence of their work.”
– Bryce Bartmann, Chief Digital Expertise Advisor, Shell
In conclusion, the Python Knowledge Supply API for Apache Spark™ is a robust addition that addresses important challenges beforehand confronted by knowledge engineers working with advanced knowledge sources and sinks, notably in streaming contexts. Whether or not utilizing the “easy” or partition-aware API, engineers now have the instruments to combine a broader array of information sources and sinks into their Spark pipelines effectively. As our walkthrough and the instance code demonstrated, implementing and utilizing this API is easy, enabling fast wins for predictive upkeep and different use instances. The Databricks documentation (and the Open Supply documentation) clarify the API in additional element, and several other Python knowledge supply examples might be discovered right here.
Lastly, the emphasis on creating customized knowledge sources as modular, reusable elements can’t be overstated. By abstracting these knowledge sources into standalone libraries, groups can foster a tradition of code reuse and collaboration, additional enhancing productiveness and innovation. As we proceed to discover and push the boundaries of what is doable with massive knowledge and IoT, applied sciences just like the Python Knowledge Supply API will play a pivotal position in shaping the way forward for data-driven decision-making within the power sector and past.
If you’re already a Databricks buyer, seize and modify one in all these examples to unlock your knowledge that’s sitting behind a REST API. If you’re not but a Databricks buyer, get began free of charge and check out one of many examples at present.