Introduction
Think about having a private assistant that not solely understands your requests but in addition is aware of precisely how one can execute them, whether or not it’s performing a fast calculation or fetching the newest inventory market information. On this article, we delve into the fascinating world of AI brokers, exploring how one can construct your individual utilizing the LlamaIndex framework. We’ll information you step-by-step by way of creating these clever brokers, highlighting the facility of LLM‘s function-calling capabilities, and demonstrating how they’ll make choices and perform duties with spectacular effectivity. Whether or not you’re new to AI or an skilled developer, this information will present you how one can unlock the total potential of AI brokers in just some traces of code.
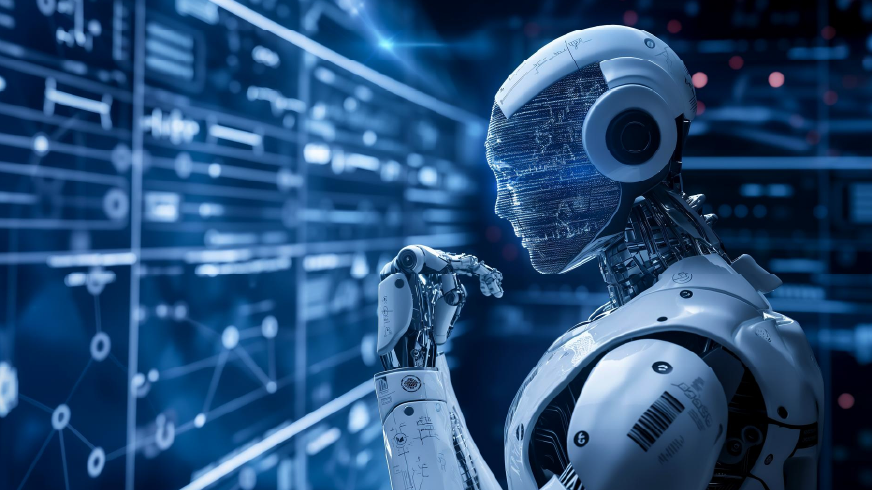
Studying Outcomes
- Perceive the fundamentals of AI brokers and their problem-solving capabilities.
- Discover ways to implement AI brokers utilizing the LlamaIndex framework.
- Discover the function-calling options in LLMs for environment friendly process execution.
- Uncover how one can combine net search instruments inside your AI brokers.
- Acquire hands-on expertise in constructing and customizing AI brokers with Python.
This text was revealed as part of the Information Science Blogathon.
What are AI Brokers?
AI brokers are like digital assistants on steroids. They don’t simply reply to your instructions—they perceive, analyze, and make choices on one of the simplest ways to execute these instructions. Whether or not it’s answering questions, performing calculations, or fetching the newest information, AI brokers are designed to deal with complicated duties with minimal human intervention. These brokers can course of pure language queries, establish the important thing particulars, and use their skills to supply probably the most correct and useful responses.
Why Use AI Brokers?
The rise of AI brokers is reworking how we work together with know-how. They will automate repetitive duties, improve decision-making, and supply customized experiences, making them invaluable in numerous industries. Whether or not you’re in finance, healthcare, or e-commerce, AI brokers can streamline operations, enhance customer support, and supply deep insights by dealing with duties that will in any other case require vital guide effort.
What’s LlamaIndex?
LlamaIndex is a cutting-edge framework designed to simplify the method of constructing AI brokers utilizing Giant Language Fashions (LLMs). It leverages the facility of LLMs like OpenAI’s fashions, enabling builders to create clever brokers with minimal coding. With LlamaIndex, you may plug in customized Python features, and the framework will robotically combine these with the LLM, permitting your AI agent to carry out a variety of duties.
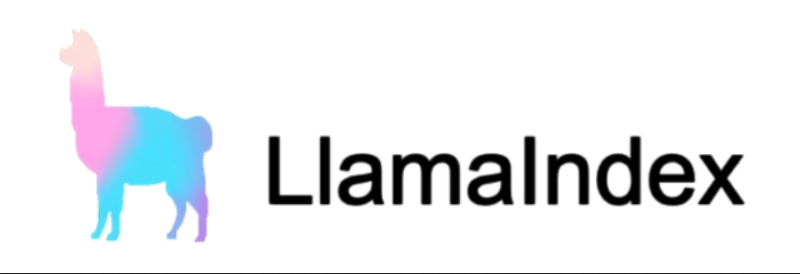
Key Options of LlamaIndex
- Operate Calling: LlamaIndex permits AI brokers to name particular features based mostly on person queries. This characteristic is important for creating brokers that may deal with a number of duties.
- Software Integration: The framework helps the combination of varied instruments, together with net search, knowledge evaluation, and extra, enabling your agent to carry out complicated operations.
- Ease of Use: LlamaIndex is designed to be user-friendly, making it accessible to each freshmen and skilled builders.
- Customizability: With help for customized features and superior options like pydantic fashions, LlamaIndex supplies the pliability wanted for specialised purposes.
Steps to Implement AI Brokers Utilizing LlamaIndex
Allow us to now look onto the steps on how we are able to implement AI brokers utilizing LlamaIndex.
Right here we will probably be utilizing GPT-4o from OpenAI as our LLM mannequin, and querying the online is being carried out utilizing Bing search. Llama Index already has Bing search instrument integration, and it may be put in with this command.
!pip set up llama-index-tools-bing-search
Step1: Get the API key
First it’s essential create a Bing search API key, which might be obtained by making a Bing useful resource from the under hyperlink. For experimentation, Bing additionally supplies a free tier with 3 calls per second and 1k calls monthly.
Step2: Set up the Required Libraries
Set up the mandatory Python libraries utilizing the next instructions:
%%seize
!pip set up llama_index llama-index-core llama-index-llms-openai
!pip set up llama-index-tools-bing-search
Step3: Set the Setting Variables
Subsequent, set your API keys as surroundings variables in order that LlamaIndex can entry them throughout execution.
import os
os.environ["OPENAI_API_KEY"] = "sk-proj-"
os.environ['BING_API_KEY'] = ""
Step4: Initialize the LLM
Initialize the LLM mannequin (on this case, GPT-4o from OpenAI) and run a easy check to substantiate it’s working.
from llama_index.llms.openai import OpenAI
llm = OpenAI(mannequin="gpt-4o")
llm.full("1+1=")
Step5: Create Two Completely different Features
Create two features that your AI agent will use. The primary operate performs a easy addition, whereas the second retrieves the newest inventory market information utilizing Bing Search.
from llama_index.instruments.bing_search import BingSearchToolSpec
def addition_tool(a:int, b:int) -> int:
"""Returns sum of inputs"""
return a + b
def web_search_tool(question:str) -> str:
"""An internet question instrument to retrieve newest inventory information"""
bing_tool = BingSearchToolSpec(api_key=os.getenv('BING_API_KEY'))
response = bing_tool.bing_news_search(question=question)
return response
For a greater operate definition, we are able to additionally make use of pydantic fashions. However for the sake of simplicity, right here we’ll depend on LLM’s means to extract arguments from the person question.
Step6: Create Operate Software Object from Person-defined Features
from llama_index.core.instruments import FunctionTool
add_tool = FunctionTool.from_defaults(fn=addition_tool)
search_tool = FunctionTool.from_defaults(fn=web_search_tool)
A operate instrument permits customers to simply convert any user-defined operate right into a instrument object.
Right here, the operate identify is the instrument identify, and the doc string will probably be handled as the outline, however this may also be overridden like under.
instrument = FunctionTool.from_defaults(addition_tool, identify="...", description="...")
Step7: Name predict_and_call technique with person’s question
question = "what's the present market worth of apple"
response = llm.predict_and_call(
instruments=[add_tool, search_tool],
user_msg=question, verbose = True
)
Right here we’ll name llm’s predict_and_call technique together with the person’s question and the instruments we outlined above. Instruments arguments can take a couple of operate by putting all features inside a listing. The tactic will undergo the person’s question and determine which is probably the most appropriate instrument to carry out the given process from the checklist of instruments.
Pattern output
=== Calling Operate ===
Calling operate: web_search_tool with args: {"question": "present market worth of Apple inventory"}
=== Operate Output ===
[['Warren Buffett Just Sold a Huge Chunk of Apple Stock. Should You Do the Same?', ..........
Step8: Putting All Together
from llama_index.llms.openai import OpenAI
from llama_index.tools.bing_search import BingSearchToolSpec
from llama_index.core.tools import FunctionTool
llm = OpenAI(model="gpt-4o")
def addition_tool(a:int, b:int)->int:
"""Returns sum of inputs"""
return a + b
def web_search_tool(query:str) -> str:
"""A web query tool to retrieve latest stock news"""
bing_tool = BingSearchToolSpec(api_key=os.getenv('BING_API_KEY'))
response = bing_tool.bing_news_search(query=query)
return response
add_tool = FunctionTool.from_defaults(fn=addition_tool)
search_tool = FunctionTool.from_defaults(fn=web_search_tool)
query = "what is the current market price of apple"
response = llm.predict_and_call(
tools=[add_tool, search_tool],
user_msg=question, verbose = True
)
Superior Customization
For these trying to push the boundaries of what AI brokers can do, superior customization provides the instruments and methods to refine and develop their capabilities, permitting your agent to deal with extra complicated duties and ship much more exact outcomes.
Enhancing Operate Definitions
To enhance how the AI agent interprets and makes use of features, you may incorporate pydantic fashions. This provides sort checking and validation, making certain that your agent processes inputs accurately.
Dealing with Complicated Queries
For extra complicated person queries, take into account creating further instruments or refining current ones to deal with a number of duties or extra intricate requests. This would possibly contain including error dealing with, logging, and even customized logic to handle how the agent responds to totally different eventualities.
Conclusion
AI brokers can course of person inputs, cause about one of the best strategy, entry related data, and execute actions to supply correct and useful responses. They will extract parameters specified within the person’s question and cross them to the related operate to hold out the duty. With LLM frameworks equivalent to LlamaIndex, Langchain, and many others., one can simply implement brokers with a couple of traces of code and in addition customise issues equivalent to operate definitions utilizing pydantic fashions.
Key Takeaways
- Brokers can take a number of unbiased features and decide which operate to execute based mostly on the person’s question.
- With Operate Calling, LLM will determine one of the best operate to finish the duty based mostly on the operate identify and the outline.
- Operate identify and outline might be overridden by explicitly specifying the operate identify and outline parameter whereas creating the instrument object.
- Llamaindex has in-built instruments and methods to implement AI brokers in a couple of traces of code.
- It’s additionally price noting that function-calling brokers might be carried out solely utilizing LLMs that help function-calling.
Ceaselessly Requested Questions
A. An AI agent is a digital assistant that processes person queries, determines one of the best strategy, and executes duties to supply correct responses.
A. LlamaIndex is a well-liked framework that permits straightforward implementation of AI brokers utilizing LLMs, like OpenAI’s fashions.
A. Operate calling permits the AI agent to pick probably the most acceptable operate based mostly on the person’s question, making the method extra environment friendly.
A. You’ll be able to combine net search by utilizing instruments like BingSearchToolSpec, which retrieves real-time knowledge based mostly on queries.
A. Sure, AI brokers can consider a number of features and select one of the best one to execute based mostly on the person’s request.
The media proven on this article is just not owned by Analytics Vidhya and is used on the Creator’s discretion.