Whether or not you are getting began along with your customized NetSuite deployment, or simply utilizing NetSuite to run your AP course of, one of the crucial frequent duties you will do is creating vendor payments.
Manually creating vendor payments could be a ache – the NetSuite UI is just not very simple, and there are hidden complexities (and a few actually cool options) which are current solely within the API and never on the NetSuite app.
Nonetheless, the complexity of the NetSuite API may be overwhelming. It takes a variety of devoted time (and energy) to arrange an API integration and really automate your NetSuite workflows.
That is the place Nanonets is available in, offering a plug-and-play integration with NetSuite that eliminates the effort and confusion, making vendor invoice automation easy.
Nanonets automates vendor invoice entry into NetSuite, and units up seamless 2-way and 3-way matching in lower than quarter-hour!
However earlier than we get too far forward of ourselves, let’s first perceive how you can arrange the NetSuite API and create vendor payments with ease.
Understanding Vendor Payments in NetSuite
A Vendor Invoice is a report used to trace the prices incurred when buying services or products from a provider. In NetSuite, vendor payments are essential for monetary and accounting functions, serving to companies streamline funds and handle stock successfully. These data additionally function a supply for monitoring excellent quantities attributable to suppliers.
Relying on the character of your transactions, it’s possible you’ll must create several types of vendor payments.
- Expense-based Vendor Payments: These payments are used for recording basic bills like utilities, lease, or companies. No bodily objects are related to these payments.
- Merchandise-based Vendor Payments: These payments contain tangible items or stock bought from the seller. This kind of invoice can embrace line objects for every services or products.
Moreover, each Expense-based and Merchandise-based Vendor payments may be categorised into beneath 2 essential teams relying on whether or not they’re linked to a purchase order order or not.
- Standalone Vendor Payments: These payments are impartial and never linked to any Buy Orders (POs). They’re usually used for ad-hoc purchases or companies.
- Vendor Payments Matching Buy Orders: These payments correspond to present POs, guaranteeing that the billing matches what was ordered.
Organising the NetSuite API
Earlier than you can begin creating vendor payments utilizing the NetSuite API, you will must arrange your account entry and guarantee correct authentication. Right here’s how you are able to do it:
Receive Account Credentials:
- Log in to your NetSuite account.
- Navigate to Setup > Firm > Allow Options.
- Below the SuiteCloud tab, make sure that SOAP Net Providers and Token-Primarily based Authentication are enabled.
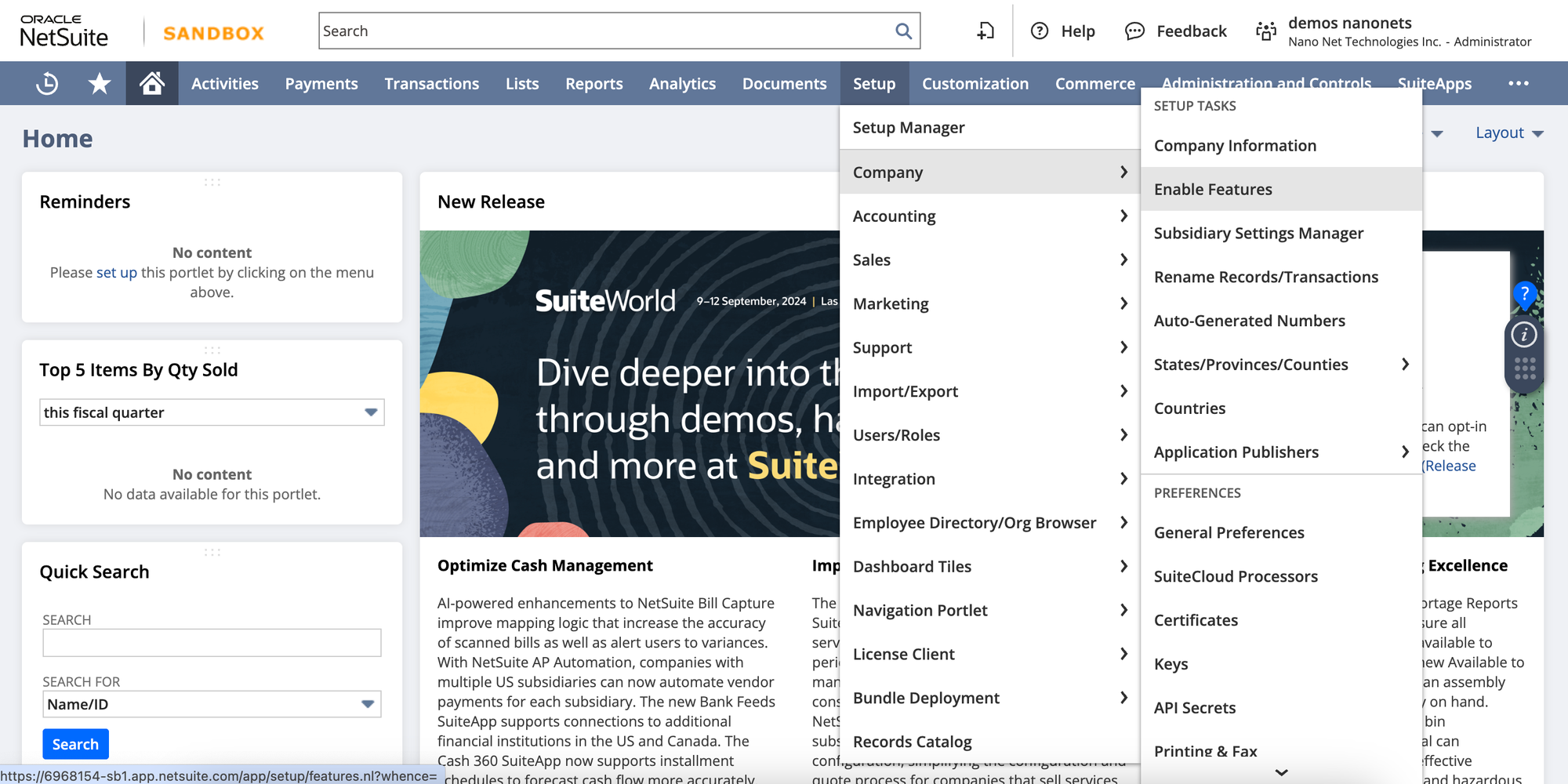
Create an Integration Report:
- Go to Setup > Integration > Handle Integrations > New.
- Fill out the required fields, and observe down the Client Key and Client Secret supplied.
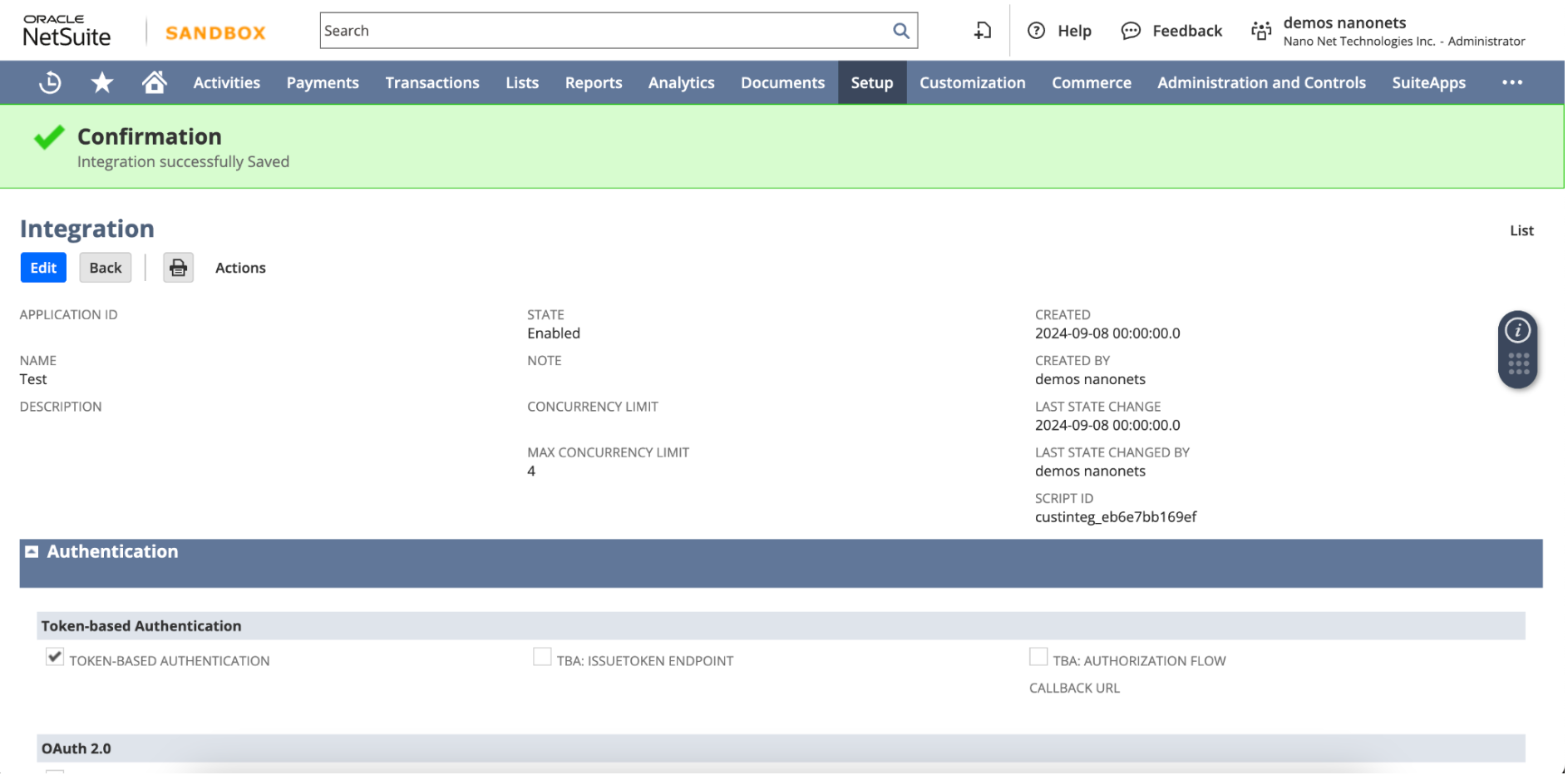
Set Up Token-Primarily based Authentication (TBA):
- Navigate to Setup > Customers/Roles > Entry Tokens > New.
- Choose the mixing report you created, and generate the Token ID and Token Secret.
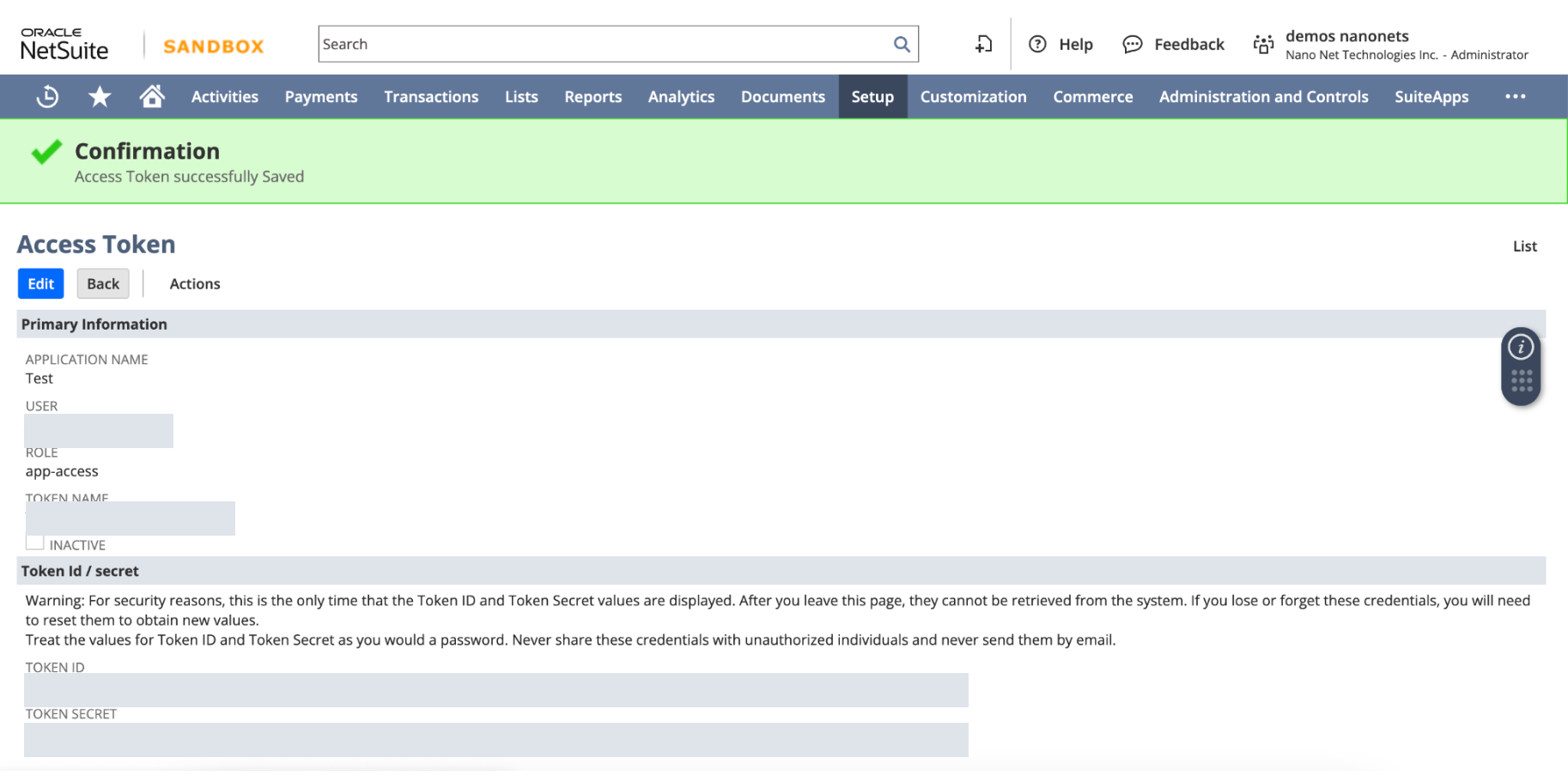
Along with your Account ID, Client Key, Client Secret, Token ID, and Token Secret, you are now able to make authenticated API calls to NetSuite.
Making a Vendor Invoice in NetSuite
NetSuite API lets you create Vendor Payments programmatically, guaranteeing seamless integration between programs. Under is a step-by-step information to making a Vendor Invoice utilizing NetSuite’s REST API in python.
Let’s dive into creating each item-based and expense-based vendor payments utilizing Python and NetSuite’s REST API.
Authentication
Earlier than making any API calls to NetSuite, it’s essential to authenticate utilizing OAuth 1.0. The next Python code makes use of the requests_oauthlib
library to authenticate the request utilizing your consumer_key
, consumer_secret
, token_key
, and token_secret
.
This is the way you authenticate utilizing OAuth 1.0:
import requests
from requests_oauthlib import OAuth1
# Authentication particulars
auth = OAuth1('consumer_key', 'consumer_secret', 'token_key', 'token_secret')
When you’re authenticated, you are able to construct your payloads.
Create Vendor Invoice Payload
1. Merchandise-Primarily based Vendor Invoice
When you’re billing for particular services or products, an item-based vendor invoice is right. This is the payload construction for an item-based invoice:
# Merchandise-based Vendor Invoice payload
item_payload = {
"entity": {"id": "1234"}, # Vendor ID
"trandate": "2024-09-01", # Transaction Date
"duedate": "2024-09-15", # Due Date
"foreign money": {"id": "1"}, # Forex ID (USD)
"phrases": {"id": "1"}, # Fee phrases
"merchandise": [
{
"item": {"id": "5678"}, # Item ID
"quantity": 10, # Quantity of the item
"rate": 20.00 # Unit price of the item
}
],
"memo": "Vendor Invoice for workplace provides"
}
2. Expense-Primarily based Vendor Invoice
For prices similar to lease, utilities, or consulting charges, an expense-based vendor invoice is extra acceptable. This is the payload for an expense-based invoice:
# Expense-based Vendor Invoice payload
expense_payload = {
"entity": {"id": "5678"}, # Vendor ID
"trandate": "2024-09-01", # Transaction Date
"duedate": "2024-09-15", # Due Date
"foreign money": {"id": "1"}, # Forex ID (USD)
"phrases": {"id": "1"}, # Fee phrases
"expense": [
{
"account": {"id": "4000"}, # Expense account ID
"amount": 500.00, # Amount of the expense
"memo": "Consulting services for August" # Memo for the expense
}
],
"memo": "Vendor Invoice for consulting companies"
}
Ship POST Request
As soon as the payload is created, the subsequent step is to ship a POST request to the NetSuite API utilizing the authenticated session. Under is the perform to deal with the request:
def create_vendor_bill(auth, payload):
url = "https://.suitetalk.api.netsuite.com/companies/relaxation/report/v1/vendorBill"
headers = {"Content material-Sort": "utility/json"}
# Ship POST request to create the seller invoice
response = requests.put up(url, json=payload, headers=headers, auth=auth)
return response
# Ship the seller invoice creation request
response = create_vendor_bill(auth, payload)
URL: That is the NetSuite REST API endpoint for creating vendor payments. Change
along with your precise NetSuite account ID.
Response Dealing with
As soon as the POST request is made, the API returns a response. It’s best to test the standing of the response and deal with it accordingly. If the invoice is created efficiently, the response will embrace particulars such because the Vendor Invoice ID and standing.
# Response Dealing with
if response.status_code == 200:
print("Vendor Invoice created efficiently:", response.json())
else:
print("Error creating Vendor Invoice:", response.status_code, response.textual content)
Instance of a Profitable Response
If the Vendor Invoice is efficiently created, the response could appear like this:

As soon as the invoice is created efficiently it’ll present up in NetSuite dashboard just like the beneath instance.
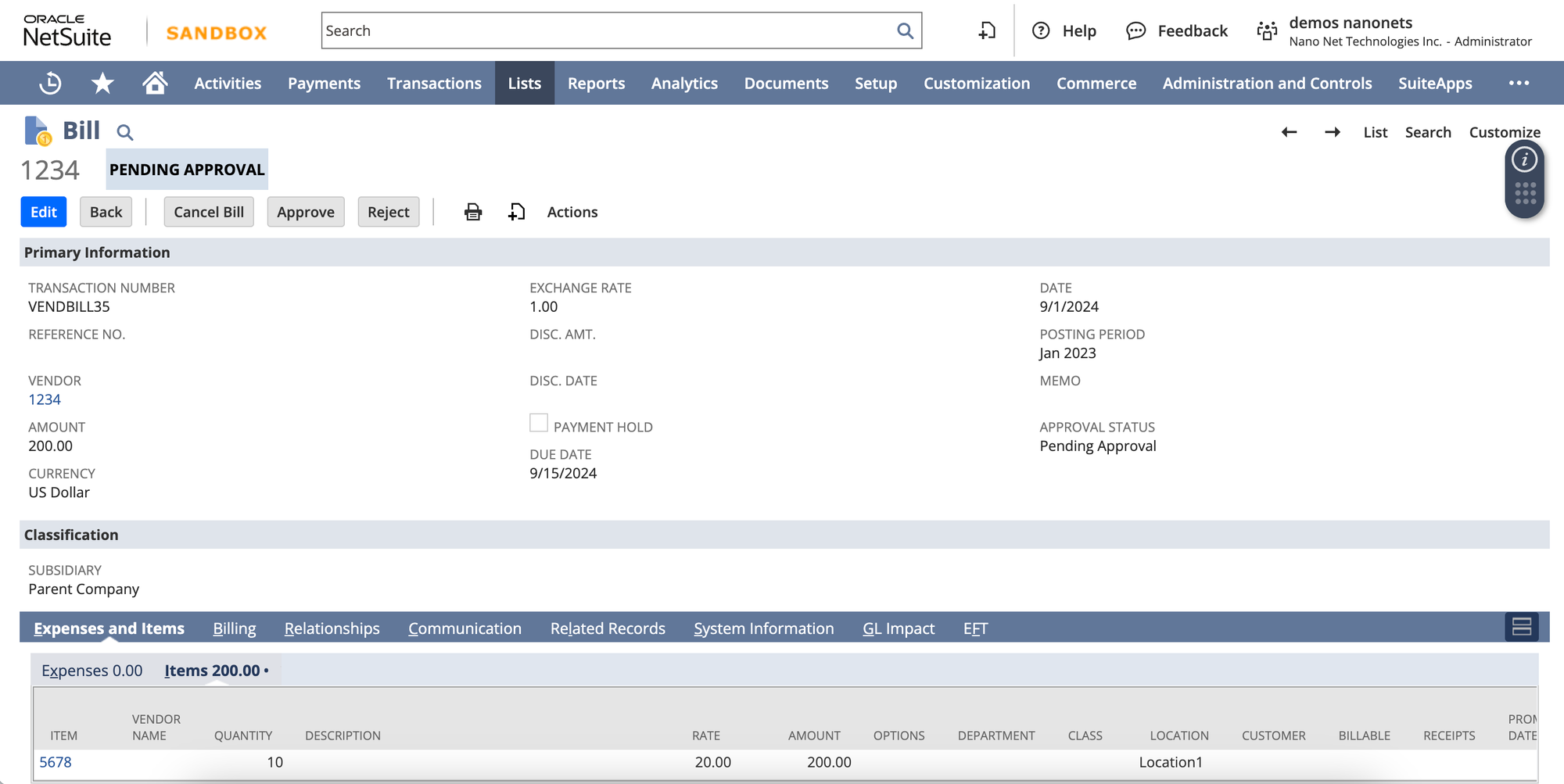
Obtain Full Code:
The core code for posting a vendor invoice utilizing NetSuite stays constant. Nonetheless, the payload varies relying on the kind of vendor invoice being added. Let’s discover how you can ship a POST request so as to add numerous sorts of vendor payments utilizing the NetSuite API.
The right way to Retrieve Inside IDs in NetSuite
To populate the mandatory fields within the payloads (similar to itemID
, currencyID
, termsID
, and accountID
), you will want to make use of NetSuite’s Inside IDs. These IDs correspond to particular entities, objects, or phrases inside your NetSuite account.
You’ll find these inner IDs by way of the next steps:
- NetSuite UI: Navigate to the related report (e.g., Vendor, Merchandise, or Account) within the NetSuite dashboard and allow “Inside IDs” beneath
Dwelling -> Set Preferences -> Normal -> Defaults -> Present Inside IDs
.
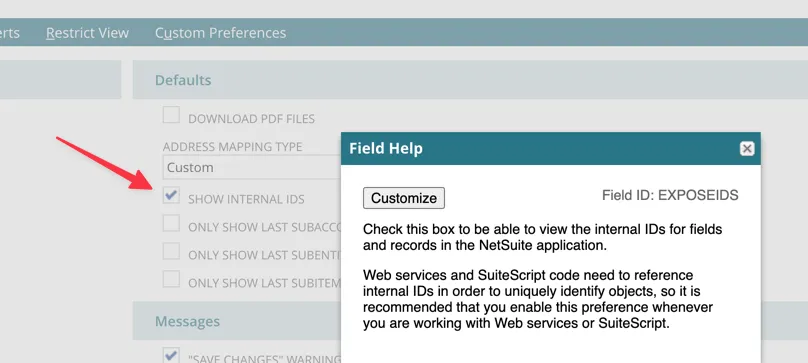
- Saved Searches: Navigate to Reviews -> Saved Searches -> All Saved Searches within the NetSuite dashboard. Create a brand new search and choose the related report sort (e.g., Gadgets, Distributors). Within the Outcomes tab, add the Inside ID discipline as a column. Working the search will show the interior IDs of the chosen data in your outcomes.
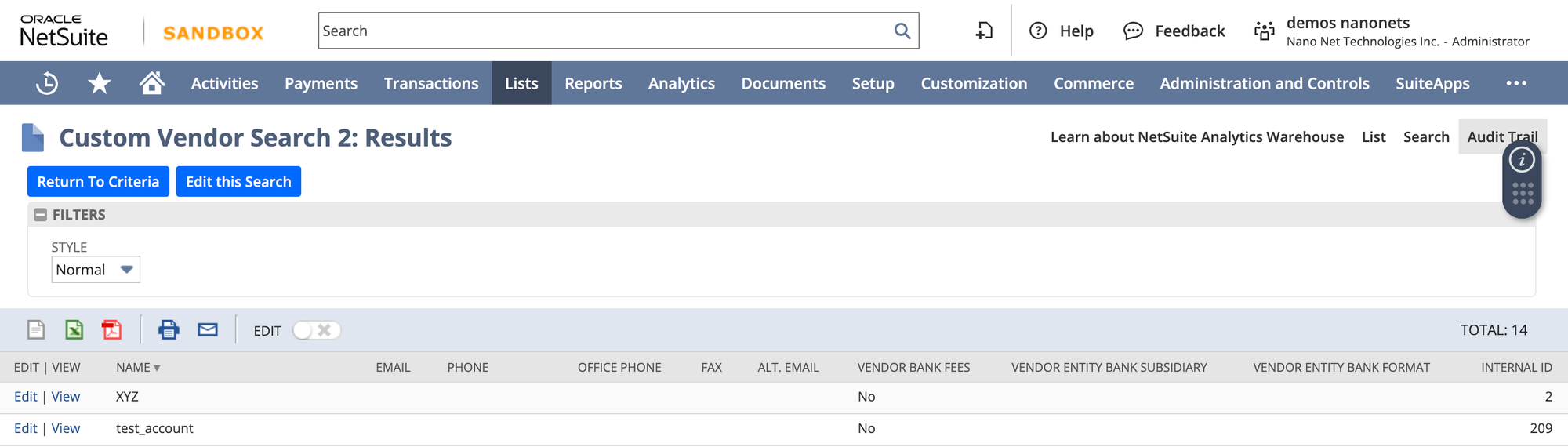
- SuiteScript: You may write and deploy a SuiteScript that retrieves inner IDs from data. After deploying the script, you may run it to entry inner IDs to be used in your API calls. Under an instance of a easy SuiteScript to retrieve inner IDs of distributors:
/**
* @NApiVersion 2.x
* @NScriptType Suitelet
*/
outline(['N/search'], perform(search) {
perform onRequest(context) {
var vendorSearch = search.create({
sort: search.Sort.VENDOR,
columns: ['internalid', 'entityid'] // inner ID and Vendor identify
});
var searchResult = vendorSearch.run();
var distributors = [];
searchResult.every(perform(outcome) {
distributors.push({
internalId: outcome.getValue({ identify: 'internalid' }),
vendorName: outcome.getValue({ identify: 'entityid' })
});
return true; // proceed iteration
});
// Ship again response as JSON
context.response.write(JSON.stringify(distributors));
}
return {
onRequest: onRequest
};
});
With these inner IDs, you may guarantee your API calls goal the right data, enhancing accuracy and effectivity in your vendor invoice creation course of.
Vendor Invoice Payloads for Totally different Situations in NetSuite
When creating vendor payments in NetSuite, the kind of expense you are dealing with can range, from a number of line objects to recurring payments or making use of reductions or credit. Under are JSON payload examples for these totally different eventualities.
Vendor Payments with A number of Line Gadgets
Vendor payments with a number of line lets you specify a number of objects, every with its personal amount and charge, and ensures that every merchandise is recorded precisely.
Merchandise-Primarily based Vendor Invoice Payload:
# Merchandise-based Vendor Invoice with a number of line objects
item_payload = {
"entity": {"id": "vendor_id"},
"trandate": "2024-09-01", # Transaction Date
"account": {"id": "account_id"}, # Account ID
"objects": [
{
"item": {"id": "item_id_1"}, # Item 1 ID
"quantity": 5,
"rate": 50.00 # Unit price for Item 1
},
{
"item": {"id": "item_id_2"}, # Item 2 ID
"quantity": 3,
"rate": 150.00 # Unit price for Item 2
}
]
}
Expense-Primarily based Vendor Invoice Payload:
# Expense-based Vendor Invoice with a number of line objects
expense_payload = {
"entity": {"id": "vendor_id"},
"trandate": "2024-09-01", # Transaction Date
"account": {"id": "expense_account_id"}, # Expense account ID
"expense": [
{
"account": {"id": "expense_account_1"}, # Expense account 1
"amount": 250.00, # Amount for first expense
"memo": "Consulting services"
},
{
"account": {"id": "expense_account_2"}, # Expense account 2
"amount": 450.00, # Amount for second expense
"memo": "Legal services"
}
]
}
This payload construction lets you add a number of line objects or bills in a single vendor invoice.
Recurring Vendor Payments
Recurring vendor payments are perfect for dealing with common, predictable bills similar to subscriptions or month-to-month companies. The recurrence possibility ensures the invoice generates robotically till a specified finish date.
Merchandise-Primarily based Recurring Vendor Invoice Payload:
# Merchandise-based Recurring Vendor Invoice payload
item_payload = {
"entity": {"id": "vendor_id"},
"tranDate": "2024-09-01", # Begin Date
"account": {"id": "account_id"}, # Account ID
"merchandise": [
{
"item": {"id": "recurring_item_id"}, # Item ID
"quantity": 1,
"rate": 500.00 # Rate for the item
}
],
"recurrence": {
"frequency": "month-to-month", # Recurring frequency
"endDate": "2024-12-01" # Finish date of recurrence
}
}
Expense-Primarily based Recurring Vendor Invoice Payload:
# Expense-based Recurring Vendor Invoice payload
expense_payload = {
"entity": {"id": "vendor_id"},
"tranDate": "2024-09-01", # Begin Date
"account": {"id": "expense_account_id"}, # Expense account ID
"expense": [
{
"account": {"id": "recurring_expense_account_id"}, # Recurring expense account
"amount": 600.00, # Amount for the recurring expense
"memo": "Monthly software subscription"
}
],
"recurrence": {
"frequency": "month-to-month", # Recurring frequency
"endDate": "2024-12-01" # Finish date of recurrence
}
}
This ensures that vendor payments are generated every month till the tip date, decreasing the necessity for handbook entries.
Making use of Reductions or Credit to Vendor Payments
In instances the place a vendor presents a reduction or credit score, you may simply mirror this in your vendor invoice. Under are the payloads for each item-based and expense-based vendor payments with utilized reductions.
Merchandise-Primarily based Vendor Invoice with Low cost Payload:
# Merchandise-based Vendor Invoice with low cost
item_payload = {
"entity": {"id": "vendor_id"},
"tranDate": "2024-09-01", # Transaction Date
"account": {"id": "account_id"}, # Account ID
"merchandise": [
{
"item": {"id": "item_id"}, # Item ID
"quantity": 1,
"rate": 100.00, # Original rate
"discount": 10.00 # Discount applied
}
]
}
Expense-Primarily based Vendor Invoice with Low cost Payload:
# Expense-based Vendor Invoice with low cost
expense_payload = {
"entity": {"id": "vendor_id"},
"tranDate": "2024-09-01", # Transaction Date
"account": {"id": "expense_account_id"}, # Expense account ID
"expense": [
{
"account": {"id": "expense_account_id"}, # Expense account ID
"amount": 100.00, # Original amount
"memo": "Discount applied on services",
"discount": 15.00 # Discount applied
}
]
}
This setup allows you to apply vendor-specific reductions to each item-based and expense-based payments.
Making a Vendor Invoice that Matches a Buy Order
Matching a vendor invoice to a purchase order order ensures that your invoice aligns with the phrases of your PO. There are two major strategies to realize this:
Match the Invoice Line to the PO Line
This technique is right whenever you need to partially invoice the PO, matching particular line objects on the seller invoice to corresponding traces within the buy order. That is significantly helpful for advanced orders with a number of objects, the place some objects could have been acquired or invoiced earlier than others. Whenever you use line-level matching, solely the billed traces are up to date, and the PO stays partially open for future billing.
payload = {
"entity": {"id": "vendor_id"},
"tranDate": "2024-09-01",
"purchaseOrder": {"id": "purchase_order_id"},
"merchandise": [
{
"purchaseOrderLine": {"id": "po_line_id"},
"quantity": 10,
"rate": 50.00
}
]
}
🗒️
Matching Invoice line to PO line permits flexibility for future changes or extra billing towards the remaining PO traces.
Rework the PO right into a Vendor Invoice
If the acquisition order has been absolutely fulfilled and also you’re able to invoice for all objects, reworking all the PO right into a vendor invoice is a faster answer. Nonetheless, this technique absolutely closes the PO, marking it as absolutely billed. It can’t be edited later, so make sure that all objects have been acquired and accounted for earlier than continuing with this method.
payload = {
"entity": {"id": "vendor_id"},
"tranDate": "2024-09-01",
"purchaseOrder": {"id": "purchase_order_id"}
}
🗒️
When reworking a PO right into a vendor invoice, the PO is locked and marked as absolutely billed. Use this technique rigorously to keep away from prematurely closing a PO that will require extra changes.
Widespread Pitfalls and Troubleshooting
Creating vendor payments by way of the NetSuite API is a robust solution to automate your monetary processes, nevertheless it comes with its challenges. Listed below are some frequent pitfalls and how you can keep away from them:
Pitfall | Answer |
---|---|
Authentication Points | Double-check your tokens, keys, and permissions. Make sure that Token-Primarily based Authentication (TBA) is appropriately arrange. |
Lacking or Incorrect Fields | All the time consult with the NetSuite API documentation to make sure all required fields are included and appropriately formatted. |
Information Synchronization Points | Implement common GET queries to confirm that the information in your system matches what’s in NetSuite. Think about using middleware or an integration platform to take care of synchronization. |
Price Limits | Monitor your API utilization and implement methods like request batching or throttling to remain inside limits. |
Dealing with Partial Exports | Implement error dealing with and logging to determine and deal with partial exports promptly. |
Create Vendor Invoice on Netsuite utilizing Nanonets
Nanonets simplifies the method by dealing with the complexities of API authentication, scope administration, and error dealing with, making it an superb end-to-end NetSuite automation workflow supplier. By leveraging the AI-powered Nanonets platform, you may automate all the vendor invoice creation course of in NetSuite with minimal human intervention. This ensures quicker processing instances, improved accuracy, and seamless integration along with your present workflows.
Nanonets intelligently extracts key knowledge from vendor invoices, maps it straight into NetSuite, and generates correct vendor payments. Whether or not you are coping with advanced line objects, recurring payments, or expense-based entries, Nanonets handles all of it with ease.
Right here’s a demo video exhibiting how one can arrange automated Vendor Invoice creation utilizing Nanonets in beneath 3 minutes.
Nanonet’s Netsuite setup Demo
Benefits of Utilizing Nanonets:
- Native NetSuite Connection: Handles all of the intricate particulars like API authentication and lacking fields.
- Out-of-the-Field Workflows: Simplifies duties like vendor invoice creation and PO matching.
- Correct Information Extraction: Leverages Gen AI fashions to extract knowledge from invoices and different paperwork.
- Consumer and Information Administration: Gives options like doc storage and administration and crew collaboration.
- Approval Workflows: Permits for knowledge verification inside your crew earlier than posting to NetSuite.
By automating the seller invoice creation course of with Nanonets, you may guarantee effectivity, accuracy, and scalability in your accounts payable workflow, leaving the tedious duties to AI.
For extra info, try the Nanonets documentation.
Nanonets automates vendor invoice and PO entry into NetSuite, plus units up seamless 2-way, 3-way, and 4-way matching in minutes!
Conclusion
Creating vendor payments in NetSuite utilizing the API streamlines your accounts payable course of, from dealing with a number of line objects and recurring payments to making use of reductions. By customizing payloads, companies can automate vendor invoice creation, decreasing handbook effort and errors.
However why cease at simply handbook API calls? Nanonets takes automation a step additional. By harnessing AI, Nanonets automates the extraction and enter of essential bill knowledge straight into NetSuite, saving you time and eliminating the danger of human error. Whether or not it’s essential to create a easy item-based vendor payments or handle recurring bills, Nanonets ensures the method is clean, correct, and scalable.