RAG is a complicated AI method that enhances the efficiency of LLMs by retrieving related paperwork or info from exterior sources throughout textual content era; not like conventional LLMs that rely solely on inside coaching information, RAG leverages real-time info to ship extra correct and contextually related responses. Whereas Naive RAG works very properly for easy queries, it struggles with complicated questions requiring multi-step reasoning or iterative refinement.
Studying Targets
- Perceive the important thing variations between Agentic RAG and Naive RAG.
- Acknowledge the restrictions of Naive RAG in dealing with complicated queries.
- Discover various use instances the place Agentic RAG excels in multi-step reasoning duties.
- Learn to implement Agentic RAG in Python utilizing CrewAI for clever information retrieval and summarization.
- Uncover how Agentic RAG strengthens Naive RAG’s capabilities by including decision-making brokers.
This text was printed as part of the Knowledge Science Blogathon.
Agentic RAG Strengthening Capabilities of Naive RAG
Agentic RAG is a novel hybrid method that merges the strengths of Retrieval-Augmented Technology and AI Brokers. This framework enhances era and decision-making by integrating dynamic retrieval programs (RAG) with autonomous brokers. In Agentic RAG, the retriever and generator are mixed and function inside a multi-agent framework the place brokers can request particular items of knowledge and make selections primarily based on retrieved information.
Agentic RAG vs Naive RAG
- Whereas Naive RAG focuses solely on bettering era by way of info retrieval, Agentic RAG provides a layer of decision-making by way of autonomous brokers.
- In Naive RAG, the retriever is passive, retrieving information solely when requested. In distinction, Agentic RAG employs brokers that actively resolve when, how, and what to retrieve.
Prime okay retrieval in Naive RAG can fail within the following eventualities:
- Summarization Questions: “Give me a abstract of this doc”.
- Comparability Questions: “Evaluate enterprise technique of PepsiCo vs Coca Cola for the final quarter of 2023”
- Multi-part Complicated Queries: “Inform me concerning the prime arguments on Retail Inflation offered within the Mint Article and inform me concerning the prime arguments on Retail inflation on Financial Occasions Article. Make a comparability desk primarily based on the collected arguments after which generate the highest conclusions primarily based on these information.”
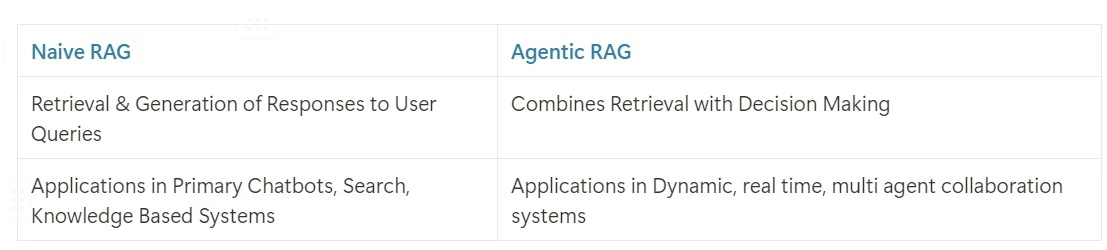
Use Instances of Agentic RAG
With the incorporation of AI brokers in RAG, agentic RAG could possibly be leveraged in a number of clever, multi-step reasoning programs. Few key use instances could possibly be the next –
- Authorized Analysis: Comparability of Authorized Paperwork and Technology of Key Clauses for fast resolution making.
- Market Evaluation: Aggressive evaluation of Prime manufacturers in a product phase.
- Medical Prognosis: Comparability of Affected person Knowledge and Newest Analysis Research to generate potential prognosis.
- Monetary Evaluation: Processing Totally different Monetary Reviews and era of key factors for higher funding insights.
- Compliance: Making certain regulatory compliance by evaluating insurance policies with legal guidelines.
Constructing Agentic RAG with Python and CrewAI
Contemplate a dataset consisting of various tech merchandise and the client points raised for these merchandise as proven within the picture under. You’ll be able to obtain the dataset from right here.
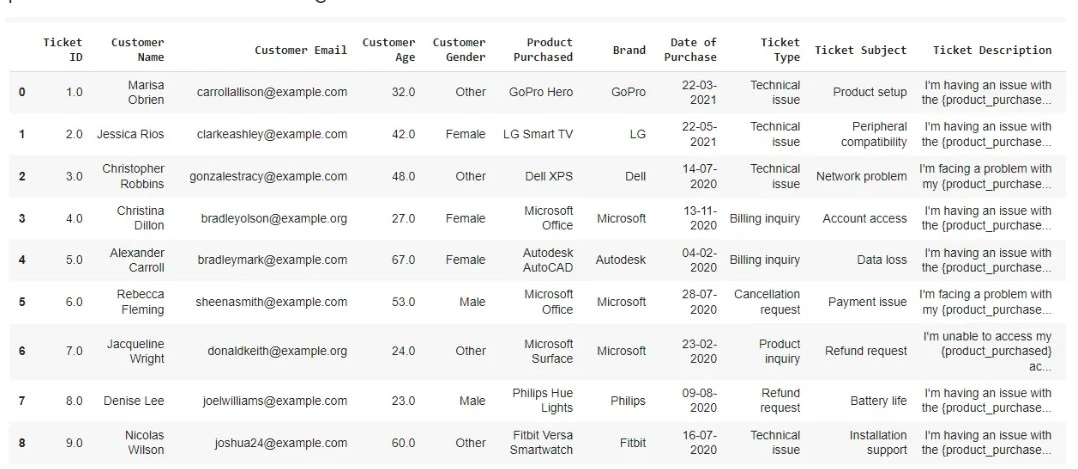
We are able to develop an agentic RAG system to summarize the highest buyer complaints for every of the manufacturers like GoPro, Microsoft and so on throughout all their merchandise. We’ll see within the following steps how we will obtain it.
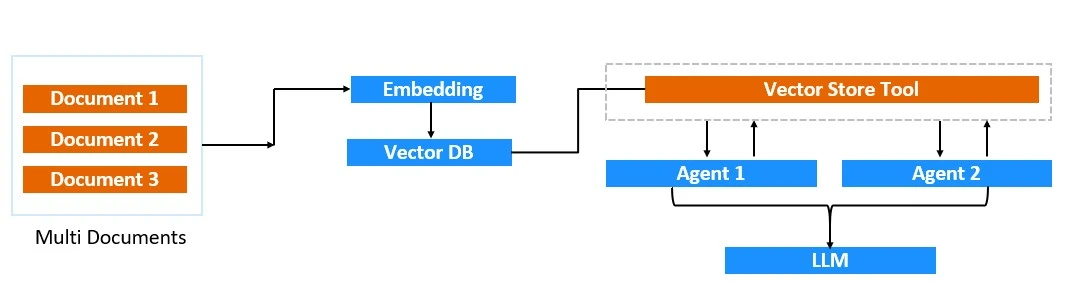
Step1: Set up Needed Python Libraries
Earlier than beginning with Agentic RAG, it’s essential to put in the required Python libraries, together with CrewAI and LlamaIndex, to assist information retrieval and agent-based duties.
!pip set up llama-index-core
!pip set up llama-index-readers-file
!pip set up llama-index-embeddings-openai
!pip set up llama-index-llms-llama-api
!pip set up 'crewai[tools]'
Step2: Import the required Python Libraries
This step entails importing important libraries to arrange the brokers and instruments for implementing Agentic RAG, enabling environment friendly information processing and retrieval.
import os
from crewai import Agent, Process, Crew, Course of
from crewai_tools import LlamaIndexTool
from llama_index.core import SimpleDirectoryReader, VectorStoreIndex
from llama_index.llms.openai import OpenAI
Step3: Learn the related csv file of Buyer Points Knowledge
Now we load the dataset containing buyer points to make it accessible for evaluation, forming the premise for retrieval and summarization.
reader = SimpleDirectoryReader(input_files=["CustomerSuppTicket_small.csv"])
docs = reader.load_data()
Step4: Outline the Open AI API key
This step units up the OpenAI API key, which is important to entry OpenAI’s language fashions for dealing with information queries.
from google.colab import userdata
openai_api_key = ''
os.environ['OPENAI_API_KEY']=openai_api_key
Step5: LLM Initialization
Initialize the Massive Language Mannequin (LLM), which can course of the question outcomes retrieved by the Agentic RAG system, enhancing summarization and insights.
llm = OpenAI(mannequin="gpt-4o")
Step6: Making a Vector Retailer Index and Question Engine
This entails making a vector retailer index and question engine, making the dataset simply searchable primarily based on similarity, with refined outcomes delivered by the LLM.
#creates a VectorStoreIndex from an inventory of paperwork (docs)
index = VectorStoreIndex.from_documents(docs)
#The vector retailer is reworked into a question engine.
#Setting similarity_top_k=5 limits the outcomes to the highest 5 paperwork which can be most much like the question,
#llm specifies that the LLM needs to be used to course of and refine the question outcomes
query_engine = index.as_query_engine(similarity_top_k=5, llm=llm)
Step7: Making a Instrument Primarily based on the Outlined Question Engine
This makes use of LlamaIndexTool to create a software primarily based on the query_engine. The software is known as “Buyer Assist Question Instrument” and is described as a technique to lookup buyer ticket information.
query_tool = LlamaIndexTool.from_query_engine(
query_engine,
identify="Buyer Assist Question Instrument",
description="Use this software to lookup the client ticket information",
)
Step8: Defining the Brokers
Brokers are outlined with particular roles and targets to carry out duties, corresponding to information evaluation and content material creation, geared toward uncovering insights from buyer information.
researcher = Agent(
position="Buyer Ticket Analyst",
objective="Uncover insights about buyer points developments",
backstory="""You're employed at a Product Firm.
Your objective is to know buyer points patterns for every of the manufacturers - 'GoPro' 'LG' 'Dell' 'Microsoft' 'Autodesk' 'Philips' 'Fitbit' 'Dyson'
'Nintendo' 'Nest' 'Sony' 'Xbox' 'Canon' 'HP' 'Amazon' 'Lenovo' 'Adobe'
'Google' 'PlayStation' 'Samsung' 'iPhone'.""",
verbose=True,
allow_delegation=False,
instruments=[query_tool],
)
author = Agent(
position="Product Content material Specialist",
objective="""Craft compelling content material on buyer points developments for every of the manufacturers - 'GoPro' 'LG' 'Dell' 'Microsoft' 'Autodesk' 'Philips' 'Fitbit' 'Dyson'
'Nintendo' 'Nest' 'Sony' 'Xbox' 'Canon' 'HP' 'Amazon' 'Lenovo' 'Adobe'
'Google' 'PlayStation' 'Samsung' 'iPhone'.""",
backstory="""You're a famend Content material Specialist, recognized on your insightful and fascinating articles.
You remodel complicated gross sales information into compelling narratives.""",
verbose=True,
allow_delegation=False,
)
The position of the ‘researcher’ agent is an analyst who will evaluate and interpret buyer assist information. The objective of this agent is outlined to “uncover insights about buyer points developments. The backstory supplies the agent with a background or context about its function. Right here, it assumes the position of a assist analyst at a product firm tasked with understanding buyer points for numerous manufacturers (e.g., GoPro, LG, Dell, and so on.). This background helps the agent give attention to every model individually because it appears for developments. The agent is supplied with the software – ‘query_tool’. Which means that the researcher agent can use this software to retrieve related buyer assist information, which it could possibly then analyze based on its objective and backstory.
The position of the ‘author’ agent is that of a content material creator centered on offering product insights. The objective of this agent is outlined to to “craft compelling content material” concerning developments in buyer points for an inventory of manufacturers. This objective will information the agent to look particularly for insights that may make good narrative or analytical content material. The backstory provides the agent further context, portray it as a extremely expert content material creator able to turning information into participating articles.
Step9: Creating the Duties for the Outlined Brokers
Duties are assigned to brokers primarily based on their roles, outlining particular tasks like information evaluation and crafting narratives on buyer points.
task1 = Process(
description="""Analyze the highest buyer points points for every of the manufacturers - 'GoPro' 'LG' 'Dell' 'Microsoft' 'Autodesk' 'Philips' 'Fitbit' 'Dyson'
'Nintendo' 'Nest' 'Sony' 'Xbox' 'Canon' 'HP' 'Amazon' 'Lenovo' 'Adobe'
'Google' 'PlayStation' 'Samsung' 'iPhone'.""",
expected_output="Detailed Buyer Points mentioning NAME of Model report with developments and insights",
agent=researcher,
)
task2 = Process(
description="""Utilizing the insights offered, develop a fascinating weblog
publish that highlights the top-customer points for every of the manufacturers - 'GoPro' 'LG' 'Dell' 'Microsoft' 'Autodesk' 'Philips' 'Fitbit' 'Dyson'
'Nintendo' 'Nest' 'Sony' 'Xbox' 'Canon' 'HP' 'Amazon' 'Lenovo' 'Adobe'
'Google' 'PlayStation' 'Samsung' 'iPhone' and their ache factors.
Your publish needs to be informative but accessible, catering to an off-the-cuff viewers.Guarantee thet the publish has NAME of the BRAND e.g. GoPro, FitBit and so on.
Make it sound cool, keep away from complicated phrases.""",
expected_output="Full weblog publish in Bullet Factors of buyer points. Guarantee thet the Weblog has NAME of the BRAND e.g. GoPro, FitBit and so on.",
agent=author,
)
Step10: Instantiating the Crew with a Sequential Course of
A crew is fashioned with brokers and duties, and this step initiates the method, the place brokers collaboratively retrieve, analyze, and current information insights.
crew = Crew(
brokers=[researcher,writer],
duties=[task1,task2],
verbose=True, # You'll be able to set it to 1 or 2 to completely different logging ranges
)
outcome = crew.kickoff()
This code creates a Crew occasion, which is a bunch of brokers assigned particular duties, after which initiates the crew’s work with the kickoff() methodology.
brokers: This parameter assigns an inventory of brokers to the crew. Right here, we’ve got two brokers: researcher and author. Every agent has a selected position—researcher focuses on analyzing the client points for every manufacturers, whereas author focuses on summarizing them.
duties: This parameter supplies an inventory of duties that the crew ought to full.
Output
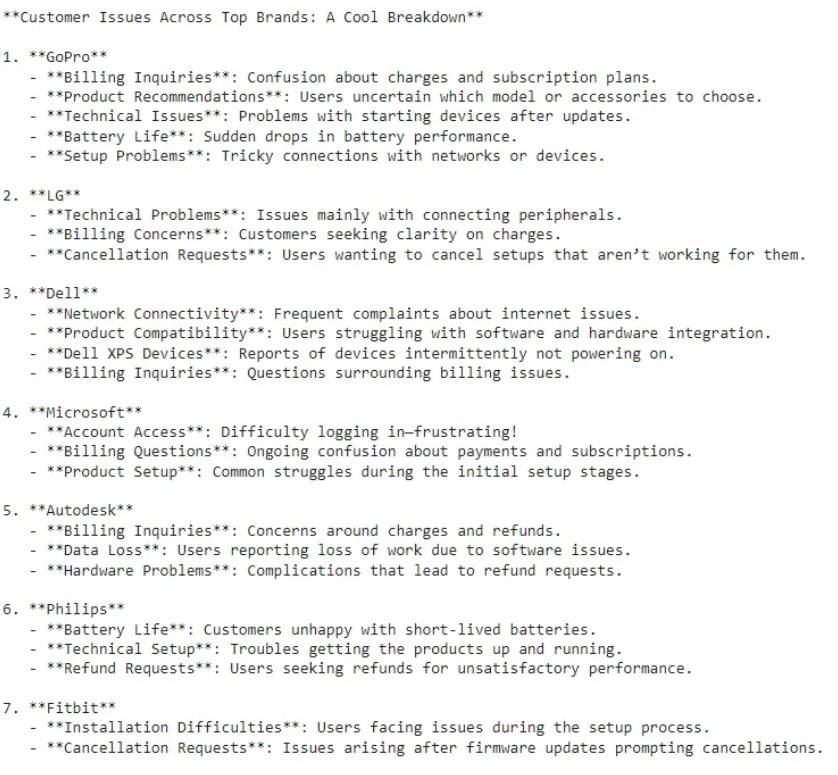
As will be seen from the output above, utilizing the Agentic RAG system, a concise abstract in bullet factors of all buyer points throughout completely different manufacturers like LG, Dell, Fitbit and so on have been generated. This concise and correct summarization of buyer points throughout the completely different manufacturers is feasible solely by way of use of the brokers.
Conclusion
Agentic RAG is a significant step ahead in Retrieval-Augmented Technology. It blends RAG’s retrieval energy with autonomous brokers’ decision-making means. This hybrid mannequin goes past Naive RAG, tackling complicated questions and comparative evaluation. Throughout industries, it supplies extra insightful, correct responses. Utilizing Python and CrewAI, builders can now create Agentic RAG programs for smarter, data-driven selections.
Key Takeaways
- Agentic RAG integrates autonomous brokers, including a layer of dynamic decision-making that goes past easy retrieval.
- Agentic RAG leverages brokers to deal with complicated queries, together with summarization, comparability, and multi-part reasoning. This functionality addresses limitations the place Naive RAG sometimes falls quick.
- Agentic RAG is efficacious in fields like authorized analysis, medical prognosis, monetary evaluation, and compliance monitoring. It supplies nuanced insights and enhanced decision-making assist.
- Utilizing CrewAI, Agentic RAG will be successfully applied in Python, demonstrating a structured method for multi-agent collaboration to deal with intricate buyer assist evaluation duties.
- Agentic RAG’s versatile agent-based structure makes it well-suited to complicated information retrieval and evaluation in various use instances, from customer support to superior analytics.
Continuously Requested Questions
A. Agentic RAG incorporates autonomous brokers that actively handle information retrieval and decision-making, whereas Naive RAG merely retrieves info on request with out further reasoning capabilities.
A. Naive RAG’s passive retrieval method is restricted to direct responses, which makes it ineffective for summarization, comparability, or multi-part queries that want iterative reasoning or layered info retrieval.
A. Agentic RAG is efficacious for duties that require multi-step reasoning, corresponding to authorized analysis, market evaluation, medical prognosis, monetary insights, and making certain compliance by way of coverage comparability.
A. Sure, you may implement Agentic RAG in Python, notably utilizing libraries like CrewAI. This helps arrange and handle brokers that collaborate to retrieve, analyze, and summarize information.
A. Industries with complicated information processing wants, corresponding to regulation, healthcare, finance, and buyer assist, stand to learn probably the most from Agentic RAG’s clever information retrieval and decision-making capabilities.
The media proven on this article just isn’t owned by Analytics Vidhya and is used on the Creator’s discretion.