Introduction
Python is broadly utilized by builders since it’s a simple language to be taught and implement. One its sturdy sides is that there are lots of samples of helpful and concise code which will assist to resolve particular issues. No matter whether or not you’re coping with recordsdata, knowledge, or internet scraping these snippets will provide help to save half of the time you’d have dedicated to typing code. Right here we’ll focus on 30 Python code by explaining them intimately with the intention to clear up daily programming issues successfully.
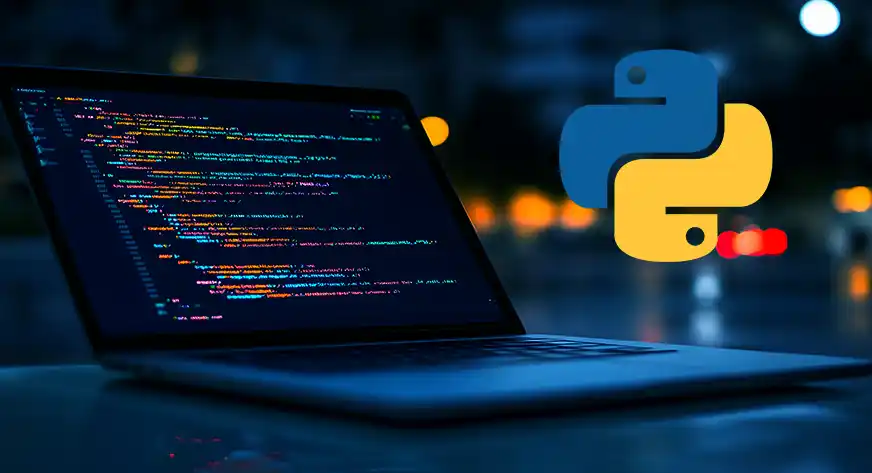
Studying Outcomes
- Study to implement widespread Python code snippets for on a regular basis duties.
- Perceive key Python ideas akin to file dealing with, string manipulation, and knowledge processing.
- Turn into aware of environment friendly Python strategies like checklist comprehensions, lambda capabilities, and dictionary operations.
- Achieve confidence in writing clear, reusable code for fixing widespread issues shortly.
Why You Ought to Use Python Code Snippets
Each programmer is aware of that Python code snippets are efficient in any venture. This manner of easily integrating new parts arrives from immediately making use of pre-written code block templates for various duties. Snippets provide help to to focus on sure venture facets with out typing monotonous code line by line. They’re most respected to be used instances like checklist processing, file I/O, and string formatting—issues you’re going to do with close to certainty in virtually any venture that you just’ll write utilizing Python.
As well as, snippets are helpful in which you could seek advice from them as you write your code, thus stopping the errors that accrue from writing comparable fundamental code again and again. That’s the reason, having realized and repeated snippets, it’s potential to realize the development of functions which might be cleaner, extra sparing of system assets, and extra sturdy.
30 Python Code Snippets for Your On a regular basis Use
Allow us to discover 30 python code snippets in your on a regular basis use under:
Studying a File Line by Line
This snippet opens a file and reads it line by line utilizing a for
loop. The with
assertion ensures the file is correctly closed after studying. strip()
removes any further whitespace or newline characters from every line.
with open('filename.txt', 'r') as file:
for line in file:
print(line.strip())
Writing to a File
We open a file for writing utilizing the w
mode. If the file doesn’t exist, Python creates it. The write()
methodology provides content material to the file. This method is environment friendly for logging or writing structured output.
with open('output.txt', 'w') as file:
file.write('Hey, World!')
Record Comprehension for Filtering
This snippet makes using checklist comprehension the place a brand new checklist is created with solely even numbers. The situation n % 2 == 0 seems for even numbers and Python creates a brand new checklist containing these numbers.
numbers = [1, 2, 3, 4, 5, 6]
even_numbers = [n for n in numbers if n % 2 == 0]
print(even_numbers)
Lambda Operate for Fast Math Operations
Lambda capabilities are these operate that are outlined as inline capabilities utilizing the lambda key phrase. On this instance, a full operate shouldn’t be outlined and a lambda operate merely provides two numbers immediately.
add = lambda x, y: x + y
print(add(5, 3))
Reversing a String
This snippet reverses a string utilizing slicing. The [::-1]
syntax slices the string with a step of -1
, which means it begins from the top and strikes backward.
string = "Python"
reversed_string = string[::-1]
print(reversed_string)
Merging Two Dictionaries
We merge two dictionaries utilizing the **
unpacking operator. This methodology is clear and environment friendly, significantly for contemporary Python variations (3.5+).
dict1 = {'a': 1, 'b': 2}
dict2 = {'c': 3, 'd': 4}
merged_dict = {**dict1, **dict2}
print(merged_dict)
Sorting a Record of Tuples
The next snippet kinds the given checklist of tuples with the assistance of dictionary key operate set to lambda operate. Specifically, the sorted() operate kind an inventory in ascending or in descending order, in accordance with the parameter handed to it; the brand new checklist was created for brand spanking new sorted values.
tuples = [(2, 'banana'), (1, 'apple'), (3, 'cherry')]
sorted_tuples = sorted(tuples, key=lambda x: x[0])
print(sorted_tuples)
Fibonacci Sequence Generator
The generator operate yields the primary n
numbers within the Fibonacci sequence. Turbines are memory-efficient as they produce gadgets on the fly reasonably than storing the complete sequence in reminiscence.
def fibonacci(n):
a, b = 0, 1
for _ in vary(n):
yield a
a, b = b, a + b
for num in fibonacci(10):
print(num)
Verify for Prime Quantity
On this snippet it’s counting that if the quantity is a chief quantity utilizing for loop from 2 to quantity sq. root. This being the case the quantity might be divided by any of the numbers on this vary, then the quantity shouldn’t be prime.
def is_prime(num):
if num < 2:
return False
for i in vary(2, int(num ** 0.5) + 1):
if num % i == 0:
return False
return True
print(is_prime(29))
Take away Duplicates from a Record
Most programming languages have a built-in operate to transform an inventory right into a set by which you get rid of duplicates as a result of units can’t let two gadgets be the identical. Lastly, the result’s transformed again to checklist from the iterable that’s obtained from utilizing the rely operate.
gadgets = [1, 2, 2, 3, 4, 4, 5]
unique_items = checklist(set(gadgets))
print(unique_items)
Easy Net Scraper with requests
and BeautifulSoup
This internet scraper makes use of Python’s ‘requests library’ the retrieves the web page and BeautifulSoup to research the returned HTML. It additionally extracts the title of the web page and prints it.
import requests
from bs4 import BeautifulSoup
response = requests.get('https://instance.com')
soup = BeautifulSoup(response.textual content, 'html.parser')
print(soup.title.textual content)
Convert Record to String
The be a part of() methodology combines parts in an inventory in to kind a string that’s delimited by a specific string (in our case it’s ‘, ‘).
gadgets = ['apple', 'banana', 'cherry']
end result=", ".be a part of(gadgets)
print(end result)
Get Present Date and Time
The datetime.now() operate return present date and time. The strftime() methodology codecs it right into a readable string format (YYYY-MM-DD HH:MM:SS).
from datetime import datetime
now = datetime.now()
print(now.strftime('%Y-%m-%d %H:%M:%S'))
Random Quantity Technology
This snippet generates a random integer between 1 and 100 utilizing the random.randint()
operate, helpful in eventualities like simulations, video games, or testing.
import random
print(random.randint(1, 100))
Flatten a Record of Lists
Record comprehension flattens an inventory of lists by iterating by every sublist and extracting the weather right into a single checklist.
list_of_lists = [[1, 2], [3, 4], [5, 6]]
flattened = [item for sublist in list_of_lists for item in sublist]
print(flattened)
Calculate Factorial Utilizing Recursion
Recursive operate calculates the factorial of a quantity by calling itself. It terminates when n
equals zero, returning 1 (the bottom case).
def factorial(n):
return 1 if n == 0 else n * factorial(n - 1)
print(factorial(5))
Swap Two Variables
Python trick lets you interchange the values saved in two variables with out utilizing a 3rd or temporary variable. It’s a easy one-liner.
a, b = 5, 10
a, b = b, a
print(a, b)
Take away Whitespace from String
The strip() is the usual methodology that, when referred to as, strips all whitespaces that seem earlier than and after a string. It’s useful when one needs to tidy up their enter knowledge earlier than evaluation.
textual content = " Hey, World! "
cleaned_text = textual content.strip()
print(cleaned_text)
Discover the Most Aspect in a Record
The max() operate find and return the largest worth inside a given checklist. It’s an efficient methodology to get the utmost of checklist of numbers, as a result of it’s quick.
numbers = [10, 20, 30, 40, 50]
max_value = max(numbers)
print(max_value)
Verify If a String is Palindrome
This snippet checks if a string is a palindrome by evaluating the string to its reverse ([::-1]
), returning True
if they’re similar.
def is_palindrome(string):
return string == string[::-1]
print(is_palindrome('madam'))
Rely Occurrences of an Aspect in a Record
The rely()
methodology returns the variety of instances a component seems in an inventory. On this instance, it counts what number of instances the quantity 3
seems.
gadgets = [1, 2, 2, 3, 3, 3, 4, 4, 4, 4]
rely = gadgets.rely(3)
print(rely)
Create a Dictionary from Two Lists
The zip()
operate pairs parts from two lists into tuples, and the dict()
constructor converts these tuples right into a dictionary. It’s a easy technique to map keys to values.
keys = ['name', 'age', 'job']
values = ['John', 28, 'Developer']
dictionary = dict(zip(keys, values))
print(dictionary)
Shuffle a Record
This snippet shuffles an inventory in place utilizing the random.shuffle()
methodology, which randomly reorders the checklist’s parts.
import random
gadgets = [1, 2, 3, 4, 5]
random.shuffle(gadgets)
print(gadgets)
Filter Parts Utilizing filter()
Filter() operate works on an inventory and takes a situation that may be a lambda operate on this case then it’ll return solely these parts of the checklist for which the situation is true (all of the even numbers right here).
numbers = [1, 2, 3, 4, 5, 6]
even_numbers = checklist(filter(lambda x: x % 2 == 0, numbers))
print(even_numbers)
Measure Execution Time of a Code Block
This snippet measures the execution time of a block of code utilizing time.time()
, which returns the present time in seconds. That is helpful for optimizing efficiency.
import time
start_time = time.time()
# Some code block
end_time = time.time()
print(f"Execution Time: {end_time - start_time} seconds")
Convert Dictionary to JSON
The json.dumps() operate take Python dictionary and returns JSON formatted string. That is particularly helpful in the case of making API requests or when storing data in a JSON object.
import json
knowledge = {'title': 'John', 'age': 30}
json_data = json.dumps(knowledge)
print(json_data)
Verify If a Key Exists in a Dictionary
This snippet checks if a key exists in a dictionary utilizing the in
key phrase. If the bottom line is discovered, it executes the code within the if
block.
particular person = {'title': 'Alice', 'age': 25}
if 'title' in particular person:
print('Key exists')
Zip A number of Lists
The zip()
operate combines a number of lists by pairing parts with the identical index. This may be helpful for creating structured knowledge from a number of lists.
names = ['Alice', 'Bob', 'Charlie']
ages = [25, 30, 35]
zipped = checklist(zip(names, ages))
print(zipped)
Generate Record of Numbers Utilizing vary()
The vary()
operate generates a sequence of numbers, which is transformed into an inventory utilizing checklist()
. On this instance, it generates numbers from 1 to 10.
numbers = checklist(vary(1, 11))
print(numbers)
Verify If a Record is Empty
This snippet checks if an inventory is empty by utilizing not
. If the checklist accommodates no parts, the situation evaluates to True
.
gadgets = []
if not gadgets:
print("Record is empty")
Finest Practices for Reusing Code Snippets
When reusing code snippets, following a number of greatest practices will assist be certain that your tasks preserve high quality and effectivity:
- Perceive Earlier than Utilizing: Keep away from blindly copying snippets. Take the time to grasp how every snippet works, its inputs, and its outputs. This may provide help to keep away from unintended bugs.
- Take a look at in Isolation: Take a look at snippets in isolation to make sure they carry out as anticipated. It’s important to confirm that the snippet works appropriately within the context of your venture.
- Remark and Doc: When modifying a snippet in your wants, at all times add feedback. Documenting modifications will provide help to or different builders perceive the logic afterward.
- Observe Coding Requirements: Make sure that snippets adhere to your coding requirements. They need to be written clearly and observe conventions like correct variable naming and formatting.
- Adapt to Your Use Case: Don’t hesitate to adapt a snippet to suit your particular venture wants. Modify it to make sure it really works optimally along with your codebase.
Creating a private library of Python code snippets is helpful and may get rid of the necessity to seek for codes in different venture. A number of instruments can be found that can assist you retailer, set up, and shortly entry your snippets:
- GitHub Gists: By use of Gists, GitHub gives an exemplary method of storing and sharing snippets of code effortlessly. These snippets might be both public or non-public in nature which makes it may be accessed from any location and gadget.
- VS Code Snippets: Visible Studio Code additionally built-in a strong snippet supervisor in which you’ll create your individual snippet units with assignable shortcuts to be utilized throughout various tasks. It additionally offers snippets the flexibility to categorize them by language.
- SnipperApp: For Mac customers, SnipperApp gives an intuitive interface to handle and categorize your code snippets, making them searchable and simply accessible when wanted.
- Elegant Textual content Snippets: Furthermore, Elegant Textual content additionally offers you the choice to grasp and even customise the snippets built-in your improvement atmosphere.
- Snippet Supervisor for Home windows: This software can help builders on home windows platforms to categorize, tag, and seek for snippets simply.
Ideas for Optimizing Snippets for Efficiency
Whereas code snippets assist streamline improvement, it’s vital to make sure that they’re optimized for efficiency. Listed below are some tricks to hold your snippets quick and environment friendly:
- Reduce Loops: If that is potential, get rid of loops and use checklist comprehensions that are sooner in Python.
- Use Constructed-in Features: Python hooked up capabilities, sum(), max() or min(), are written in C and are normally sooner than these carried out by hand.
- Keep away from International Variables: I additionally got here throughout some particular points when growing snippets, certainly one of which is the utilization of world variables, which ends up in efficiency issues. Relatively attempt to use native variables or to cross the variables as parameters.
- Environment friendly Information Buildings: When retrieving information it vital take the correct construction to finish the duty. As an example, use units to make it possible for membership test is finished in a short while whereas utilizing dictionaries to acquire fast lookups.
- Benchmark Your Snippets: Time your snippets and use debugging and profiling instruments and if potential use time it to point out you the bottlenecks. It helps in ensuring that your code shouldn’t be solely right but additionally right by way of, how briskly it will probably execute in your laptop.
Widespread Errors to Keep away from When Utilizing Python Snippets
Whereas code snippets can save time, there are widespread errors that builders ought to keep away from when reusing them:
- Copy-Pasting With out Understanding: One fault was talked about to be particularly devastating – that of lifting the code and probably not realizing what one does. This leads to an inaccurate answer when the snippet is then taken and utilized in a brand new setting.
- Ignoring Edge Instances: Snippets are good for a normal case when the standard values are entered, however they don’t contemplate particular instances (for instance, an empty area, however there could also be a lot of different values). While you write your snippets, at all times attempt to run them with totally different inputs.
- Overusing Snippets: Nonetheless, if one makes use of snippets quite a bit, he/she’s going to by no means actually get the language and simply be a Outsource Monkey, utilizing translation software program on each day foundation. Attempt to learn the way the snippets are carried out.
- Not Refactoring for Particular Wants: As one would possibly guess from their title, snippets are meant to be versatile. If a snippet shouldn’t be personalized to suit the particular venture it’s getting used on, then there’s a excessive chance that the venture will expertise some inefficiencies, or bugs it.
- Not Checking for Compatibility: Make sure that the snippet you’re utilizing is suitable with the model of Python you’re working with. Some snippets could use options or libraries which might be deprecated or version-specific.
Conclusion
These 30 Python code snippets cowl a wide selection of widespread duties and challenges that builders face of their day-to-day coding. From dealing with recordsdata, and dealing with strings, to internet scraping and knowledge processing, these snippets save time and simplify coding efforts. Hold these snippets in your toolkit, modify them in your wants, and apply them in real-world tasks to grow to be a extra environment friendly Python developer.
If you wish to be taught python without cost, right here is our introduction to python course!
Incessantly Requested Questions
A. Observe usually, discover Python documentation, and contribute to tasks on GitHub.
A. Sure, they’re straightforward to grasp and useful for freshmen in addition to skilled builders.
A. Observe by utilizing them in real-world tasks. Repetition will provide help to recall them simply.
A. Completely! These snippets present a strong basis, however you’ll be able to construct extra complicated logic round them based mostly in your wants.